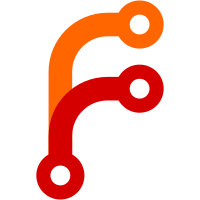
Previously neither the module nor the name of the module of pyfunctions were registered. This commit passes the module and its name when creating a new pyfunction. PyModule::add_function and PyModule::add_module have been added and are set to replace `add_wrapped` in a future release. `add_wrapped` is kept for compatibility reasons during the transition. Depending on whether a `PyModule` or `Python` is the argument for the Python function-wrapper, the module will be registered with the function.
43 lines
757 B
Rust
43 lines
757 B
Rust
//! https://github.com/PyO3/pyo3/issues/233
|
|
//!
|
|
//! The code below just tries to use the most important code generation paths
|
|
|
|
use pyo3::prelude::*;
|
|
use pyo3::wrap_pyfunction;
|
|
|
|
#[pyclass]
|
|
pub struct ModClass {
|
|
_somefield: String,
|
|
}
|
|
|
|
#[pymethods]
|
|
impl ModClass {
|
|
#[new]
|
|
fn new() -> Self {
|
|
ModClass {
|
|
_somefield: String::from("contents"),
|
|
}
|
|
}
|
|
|
|
fn noop(&self, x: usize) -> usize {
|
|
x
|
|
}
|
|
}
|
|
|
|
#[pyfunction]
|
|
fn double(x: i32) -> i32 {
|
|
x * 2
|
|
}
|
|
|
|
#[pymodule]
|
|
fn othermod(_py: Python<'_>, m: &PyModule) -> PyResult<()> {
|
|
m.add_function(wrap_pyfunction!(double))?;
|
|
|
|
m.add_class::<ModClass>()?;
|
|
|
|
m.add("USIZE_MIN", usize::min_value())?;
|
|
m.add("USIZE_MAX", usize::max_value())?;
|
|
|
|
Ok(())
|
|
}
|