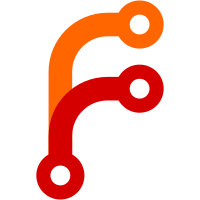
* WIP/initial routing-ish * refactor date dropdown to reuse in modal and allowe current month selection * swap linter disable line * refactor date-dropdown to return object * refactor calendar widget, add tests * change calendar start and end args to getters * refactor dashboard to use date objects instead of array of year, month * remove dashboard files for easier to follow git diff * comment out dashboard tab until route name updated * delete current tab and route * fix undefined banner time * cleanup version history serializer and upgrade data * first pass of updating tests * add changelog * update client count util test * validate end time is after start time * update comment * add current month to calendar widget * add comments for code changes to make following API update * Removed a modified file from pull request * address comments/cleanup * update variables to const * update test const * rename history -> dashboard, fix tests * fix timestamps for attribution chart * update release note * refactor using backend start and end time params * add test for adapter formatting time params * fix tests * cleanup adapter comment and query params * change back history file name for diff * rename file using cli * revert filenames * rename files via git cli * revert route file name * last cli rename * refactor mirage * hold off on running total changes * update params in test * refactor to remove conditional assertions * finish tests * fix firefox tooltip * remove current-when * refactor version history * add timezone/UTC note * final cleanup!!!! * fix test * fix client count date tests * fix date-dropdown test * clear datedropdown completely * update date selectors to accommodate new year (#18586) * Revert "hold off on running total changes" This reverts commit 8dc79a626d549df83bc47e290392a556c670f98f. * remove assumed 0 values * update average helper to only calculate for array of objects * remove passing in bar chart data, map in running totals component instead * cleanup usage stat component * clear ss filters for new queries * update csv export, add explanation to modal * update test copy * consistently return null if no upgrade during activity (instead of empty array) * update description, add clarifying comments * update tes * add more clarifying comments * fix historic single month chart * remove old test tag * Update ui/app/components/clients/dashboard.js
62 lines
2.1 KiB
JavaScript
62 lines
2.1 KiB
JavaScript
import { formatNumbers, formatTooltipNumber, calculateAverage } from 'vault/utils/chart-helpers';
|
|
import { module, test } from 'qunit';
|
|
|
|
const SMALL_NUMBERS = [0, 7, 27, 103, 999];
|
|
const LARGE_NUMBERS = {
|
|
1001: '1k',
|
|
33777: '34k',
|
|
532543: '530k',
|
|
2100100: '2.1M',
|
|
54500200100: '55B',
|
|
};
|
|
|
|
module('Unit | Utility | chart-helpers', function () {
|
|
test('formatNumbers renders number correctly', function (assert) {
|
|
assert.expect(11);
|
|
const method = formatNumbers();
|
|
assert.ok(method);
|
|
SMALL_NUMBERS.forEach(function (num) {
|
|
assert.strictEqual(formatNumbers(num), num, `Does not format small number ${num}`);
|
|
});
|
|
Object.keys(LARGE_NUMBERS).forEach(function (num) {
|
|
const expected = LARGE_NUMBERS[num];
|
|
assert.strictEqual(formatNumbers(num), expected, `Formats ${num} as ${expected}`);
|
|
});
|
|
});
|
|
|
|
test('formatTooltipNumber renders number correctly', function (assert) {
|
|
const formatted = formatTooltipNumber(120300200100);
|
|
assert.strictEqual(formatted.length, 15, 'adds punctuation at proper place for large numbers');
|
|
});
|
|
|
|
test('calculateAverage is accurate', function (assert) {
|
|
const testArray1 = [
|
|
{ label: 'foo', value: 10 },
|
|
{ label: 'bar', value: 22 },
|
|
];
|
|
const testArray2 = [
|
|
{ label: 'foo', value: undefined },
|
|
{ label: 'bar', value: 22 },
|
|
];
|
|
const testArray3 = [{ label: 'foo' }, { label: 'bar' }];
|
|
const getAverage = (array) => array.reduce((a, b) => a + b, 0) / array.length;
|
|
assert.strictEqual(calculateAverage(null), null, 'returns null if dataset it null');
|
|
assert.strictEqual(calculateAverage([]), null, 'returns null if dataset it empty array');
|
|
assert.strictEqual(
|
|
calculateAverage(testArray1, 'value'),
|
|
getAverage([10, 22]),
|
|
`returns correct average for array of objects`
|
|
);
|
|
assert.strictEqual(
|
|
calculateAverage(testArray2, 'value'),
|
|
getAverage([0, 22]),
|
|
`returns correct average for array of objects containing undefined values`
|
|
);
|
|
assert.strictEqual(
|
|
calculateAverage(testArray3, 'value'),
|
|
null,
|
|
'returns null when object key does not exist at all'
|
|
);
|
|
});
|
|
});
|