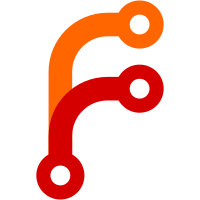
* add rotate root route * add page component * add modal * fix modal image styling * add radio buttons * add jsonToCert function to pki parser * add verify function * add verify to details route * nest rotate-root under issuer/ * copy values from old root ca * pull detail info rows into a separate component * add type declaration files * add parsing error warning to rotate root component file * add comments * add capabilities to controller * update icon * revert issuer details * refactor pki info table rows * add parsedparameters to pki helper * add alert banner * update attrs, fix info rows * add endpoint to action router * update alert banner * hide toolbar from generate root display * add download buttons to toolbar * add banner getter * fix typo in issuer details * fix assertion * move alert banner after generating root to parent * rename issuer index route file * refactor routing so model can be passed from route * add confirmLeave and done button to use existin settings done form * rename serial number to differentiate between two types * fix links, update ids to issuerId not response id * update ts declaration * change variable names add comments * update existing tests * fix comment typo * add download button test * update serializer to change subject_serial_number to serial_number for backend * remove pageTitle getter * remove old arg * round 1 of testing complete.. * finish endpoint tests * finish component tests * move toolbars to parent route * add acceptance test for rotate route * add const to hold radio button string values * remove action, fix link
53 lines
2.1 KiB
JavaScript
53 lines
2.1 KiB
JavaScript
/**
|
|
* Copyright (c) HashiCorp, Inc.
|
|
* SPDX-License-Identifier: MPL-2.0
|
|
*/
|
|
|
|
import { assert } from '@ember/debug';
|
|
import { encodePath } from 'vault/utils/path-encoding-helpers';
|
|
import ApplicationAdapter from '../application';
|
|
|
|
export default class PkiActionAdapter extends ApplicationAdapter {
|
|
namespace = 'v1';
|
|
|
|
urlForCreateRecord(modelName, snapshot) {
|
|
const { type } = snapshot.record;
|
|
const { actionType, useIssuer, issuerRef, mount } = snapshot.adapterOptions;
|
|
// if the backend mount is passed, we want that to override the URL's mount path
|
|
const backend = mount || snapshot.record.backend;
|
|
if (!backend || !actionType) {
|
|
throw new Error('URL for create record is missing required attributes');
|
|
}
|
|
const baseUrl = `${this.buildURL()}/${encodePath(backend)}`;
|
|
switch (actionType) {
|
|
case 'import':
|
|
return useIssuer ? `${baseUrl}/issuers/import/bundle` : `${baseUrl}/config/ca`;
|
|
case 'generate-root':
|
|
return useIssuer ? `${baseUrl}/issuers/generate/root/${type}` : `${baseUrl}/root/generate/${type}`;
|
|
case 'generate-csr':
|
|
return useIssuer
|
|
? `${baseUrl}/issuers/generate/intermediate/${type}`
|
|
: `${baseUrl}/intermediate/generate/${type}`;
|
|
case 'sign-intermediate':
|
|
return `${baseUrl}/issuer/${encodePath(issuerRef)}/sign-intermediate`;
|
|
case 'rotate-root':
|
|
return `${baseUrl}/root/rotate/${type}`;
|
|
default:
|
|
assert('actionType must be one of import, generate-root, generate-csr or sign-intermediate');
|
|
}
|
|
}
|
|
|
|
createRecord(store, type, snapshot) {
|
|
const serializer = store.serializerFor(type.modelName);
|
|
const url = this.urlForCreateRecord(type.modelName, snapshot);
|
|
// Send actionType as serializer requestType so that we serialize data based on the endpoint
|
|
const data = serializer.serialize(snapshot, snapshot.adapterOptions.actionType);
|
|
return this.ajax(url, 'POST', { data }).then((result) => ({
|
|
// pki/action endpoints don't correspond with a single specific entity,
|
|
// so in ember-data we'll map it to the request ID
|
|
id: result.request_id,
|
|
...result,
|
|
}));
|
|
}
|
|
}
|