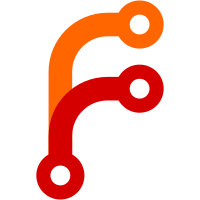
* Update browserslist * Add browserslistrc * ember-cli-update --to 3.26, fix conflicts * Run codemodes that start with ember-* * More codemods - before cp* * More codemods (curly data-test-*) * WIP ember-basic-dropdown template errors * updates ember-basic-dropdown and related deps to fix build issues * updates basic dropdown instances to new version API * updates more deps -- ember-template-lint is working again * runs no-implicit-this codemod * creates and runs no-quoteless-attributes codemod * runs angle brackets codemod * updates lint:hbs globs to only touch hbs files * removes yield only templates * creates and runs deprecated args transform * supresses lint error for invokeAction on LinkTo component * resolves remaining ambiguous path lint errors * resolves simple-unless lint errors * adds warnings for deprecated tagName arg on LinkTo components * adds warnings for remaining curly component invocation * updates global template lint rules * resolves remaining template lint errors * disables some ember specfic lint rules that target pre octane patterns * js lint fix run * resolves remaining js lint errors * fixes test run * adds npm-run-all dep * fixes test attribute issues * fixes console acceptance tests * fixes tests * adds yield only wizard/tutorial-active template * fixes more tests * attempts to fix more flaky tests * removes commented out settled in transit test * updates deprecations workflow and adds initializer to filter by version * updates flaky policies acl old test * updates to flaky transit test * bumps ember deps down to LTS version * runs linters after main merge * fixes client count tests after bad merge conflict fixes * fixes client count history test * more updates to lint config * another round of hbs lint fixes after extending stylistic rule * updates lint-staged commands * removes indent eslint rule since it seems to break things * fixes bad attribute in transform-edit-form template * test fixes * fixes enterprise tests * adds changelog * removes deprecated ember-concurrency-test-waiters dep and adds @ember/test-waiters * flaky test fix Co-authored-by: hashishaw <cshaw@hashicorp.com>
188 lines
4.1 KiB
JavaScript
188 lines
4.1 KiB
JavaScript
import { inject as service } from '@ember/service';
|
|
import { gt } from '@ember/object/computed';
|
|
import { camelize } from '@ember/string';
|
|
import Component from '@ember/component';
|
|
import { get, computed } from '@ember/object';
|
|
import layout from '../templates/components/shamir-flow';
|
|
import { A } from '@ember/array';
|
|
|
|
const pgpKeyFileDefault = () => ({ value: '' });
|
|
const DEFAULTS = {
|
|
key: null,
|
|
loading: false,
|
|
errors: A(),
|
|
threshold: null,
|
|
progress: null,
|
|
pgp_key: null,
|
|
haveSavedPGPKey: false,
|
|
started: false,
|
|
generateWithPGP: false,
|
|
pgpKeyFile: pgpKeyFileDefault(),
|
|
nonce: '',
|
|
};
|
|
|
|
export default Component.extend(DEFAULTS, {
|
|
tagName: '',
|
|
store: service(),
|
|
formText: null,
|
|
fetchOnInit: false,
|
|
buttonText: 'Submit',
|
|
thresholdPath: 'required',
|
|
generateAction: false,
|
|
layout,
|
|
|
|
init() {
|
|
this._super(...arguments);
|
|
if (this.fetchOnInit) {
|
|
this.attemptProgress();
|
|
}
|
|
},
|
|
|
|
didInsertElement() {
|
|
this._super(...arguments);
|
|
this.onUpdate(this.getProperties(Object.keys(DEFAULTS)));
|
|
},
|
|
|
|
onUpdate() {},
|
|
onLicenseError() {},
|
|
onShamirSuccess() {},
|
|
// can be overridden w/an attr
|
|
isComplete(data) {
|
|
return data.complete === true;
|
|
},
|
|
|
|
stopLoading() {
|
|
this.setProperties({
|
|
loading: false,
|
|
errors: [],
|
|
key: null,
|
|
});
|
|
},
|
|
|
|
reset() {
|
|
this.setProperties(DEFAULTS);
|
|
},
|
|
|
|
hasProgress: gt('progress', 0),
|
|
|
|
actionSuccess(resp) {
|
|
let { onUpdate, isComplete, onShamirSuccess, thresholdPath } = this;
|
|
let threshold = get(resp, thresholdPath);
|
|
let props = {
|
|
...resp,
|
|
threshold,
|
|
};
|
|
this.stopLoading();
|
|
// if we have an OTP, but update doesn't include one,
|
|
// we don't want to null it out
|
|
if (this.otp && !props.otp) {
|
|
delete props.otp;
|
|
}
|
|
this.setProperties(props);
|
|
onUpdate(props);
|
|
if (isComplete(props)) {
|
|
this.reset();
|
|
onShamirSuccess(props);
|
|
}
|
|
},
|
|
|
|
actionError(e) {
|
|
this.stopLoading();
|
|
if (e.httpStatus === 400) {
|
|
this.set('errors', e.errors);
|
|
} else {
|
|
// if licensing error, trigger parent method to handle
|
|
if (e.httpStatus === 500 && e.errors?.join(' ').includes('licensing is in an invalid state')) {
|
|
this.onLicenseError();
|
|
}
|
|
throw e;
|
|
}
|
|
},
|
|
|
|
generateStep: computed('generateWithPGP', 'haveSavedPGPKey', 'pgp_key', function () {
|
|
let { generateWithPGP, pgp_key, haveSavedPGPKey } = this;
|
|
if (!generateWithPGP && !pgp_key) {
|
|
return 'chooseMethod';
|
|
}
|
|
if (generateWithPGP) {
|
|
if (pgp_key && haveSavedPGPKey) {
|
|
return 'beginGenerationWithPGP';
|
|
} else {
|
|
return 'providePGPKey';
|
|
}
|
|
}
|
|
return '';
|
|
}),
|
|
|
|
extractData(data) {
|
|
const isGenerate = this.generateAction;
|
|
const hasStarted = this.started;
|
|
const usePGP = this.generateWithPGP;
|
|
const nonce = this.nonce;
|
|
|
|
if (!isGenerate || hasStarted) {
|
|
if (nonce) {
|
|
data.nonce = nonce;
|
|
}
|
|
return data;
|
|
}
|
|
|
|
if (usePGP) {
|
|
return {
|
|
pgp_key: data.pgp_key,
|
|
};
|
|
}
|
|
|
|
return {
|
|
attempt: data.attempt,
|
|
};
|
|
},
|
|
|
|
attemptProgress(data) {
|
|
const checkStatus = data ? false : true;
|
|
let action = this.action;
|
|
action = action && camelize(action);
|
|
this.set('loading', true);
|
|
const adapter = this.store.adapterFor('cluster');
|
|
const method = adapter[action];
|
|
|
|
method.call(adapter, data, { checkStatus }).then(
|
|
(resp) => this.actionSuccess(resp),
|
|
(...args) => this.actionError(...args)
|
|
);
|
|
},
|
|
|
|
actions: {
|
|
reset() {
|
|
this.reset();
|
|
this.set('encoded_token', null);
|
|
this.set('otp', null);
|
|
},
|
|
|
|
onSubmit(data) {
|
|
if (!data.key) {
|
|
return;
|
|
}
|
|
this.attemptProgress(this.extractData(data));
|
|
},
|
|
|
|
startGenerate(data) {
|
|
if (this.generateAction) {
|
|
data.attempt = true;
|
|
}
|
|
this.attemptProgress(this.extractData(data));
|
|
},
|
|
|
|
setKey(_, keyFile) {
|
|
this.set('pgp_key', keyFile.value);
|
|
this.set('pgpKeyFile', keyFile);
|
|
},
|
|
|
|
savePGPKey() {
|
|
if (this.pgp_key) {
|
|
this.set('haveSavedPGPKey', true);
|
|
}
|
|
},
|
|
},
|
|
});
|