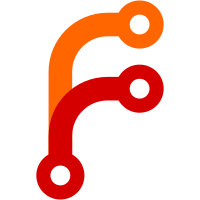
* fix issue where if dr is not enabled, the breadcrumb link did not work. Now if DR not enabled, the breadcrumb says replication and links back to rep index. * show black nav when cluster is not initialized and is loading, no need for menu items and because NavHeader component and the icon live in the app and not addons I cannot access them without moving them. I figured the black bar was enough, and it wasn't worth moving just for that * conditional change the breadcrumb link in Disaster Recovery based on what page they are currently on (details or manage). Before the breadcrumb link didn't do anything if they were on the manage page * fix slow modal loading after demoting a dr secondary. due to shamir modal not being in the addon engine and erroring out. * to prevent confusing transition state during dr demotion, set new property on cluster and compare the mode of the dr which changes from primary to secondary during demotion. If dr mode changes, showing loading status * get more specific about conditional so loader does not some on disabling, but only on demote * remove concurrency from onSubmit * revert all concurency, I think this is solved by the removal of shamir in the dom * reverse order * cleanup * forgot that tricky layout, hopefull this will fix test * remove page container, it's not needed * remove breadcrumbs if DR secondary * remove pageType no now longer using * remove conditional that is no longer hit
71 lines
2.7 KiB
JavaScript
71 lines
2.7 KiB
JavaScript
import { inject as service } from '@ember/service';
|
|
import { alias, and, equal, gte, not, or } from '@ember/object/computed';
|
|
import { get, computed } from '@ember/object';
|
|
import DS from 'ember-data';
|
|
import { fragment } from 'ember-data-model-fragments/attributes';
|
|
const { hasMany, attr } = DS;
|
|
|
|
export default DS.Model.extend({
|
|
version: service(),
|
|
|
|
nodes: hasMany('nodes', { async: false }),
|
|
name: attr('string'),
|
|
status: attr('string'),
|
|
standby: attr('boolean'),
|
|
type: attr('string'),
|
|
|
|
needsInit: computed('nodes', 'nodes.@each.initialized', function() {
|
|
// needs init if no nodes are initialized
|
|
return this.get('nodes').isEvery('initialized', false);
|
|
}),
|
|
|
|
unsealed: computed('nodes', 'nodes.{[],@each.sealed}', function() {
|
|
// unsealed if there's at least one unsealed node
|
|
return !!this.get('nodes').findBy('sealed', false);
|
|
}),
|
|
|
|
sealed: not('unsealed'),
|
|
|
|
leaderNode: computed('nodes', 'nodes.[]', function() {
|
|
const nodes = this.get('nodes');
|
|
if (nodes.get('length') === 1) {
|
|
return nodes.get('firstObject');
|
|
} else {
|
|
return nodes.findBy('isLeader');
|
|
}
|
|
}),
|
|
|
|
sealThreshold: alias('leaderNode.sealThreshold'),
|
|
sealProgress: alias('leaderNode.progress'),
|
|
sealType: alias('leaderNode.type'),
|
|
storageType: alias('leaderNode.storageType'),
|
|
hasProgress: gte('sealProgress', 1),
|
|
usingRaft: equal('storageType', 'raft'),
|
|
|
|
//replication mode - will only ever be 'unsupported'
|
|
//otherwise the particular mode will have the relevant mode attr through replication-attributes
|
|
mode: attr('string'),
|
|
allReplicationDisabled: and('{dr,performance}.replicationDisabled'),
|
|
anyReplicationEnabled: or('{dr,performance}.replicationEnabled'),
|
|
|
|
dr: fragment('replication-attributes'),
|
|
performance: fragment('replication-attributes'),
|
|
// this service exposes what mode the UI is currently viewing
|
|
// replicationAttrs will then return the relevant `replication-attributes` fragment
|
|
rm: service('replication-mode'),
|
|
drMode: alias('dr.mode'),
|
|
replicationMode: alias('rm.mode'),
|
|
replicationModeForDisplay: computed('replicationMode', function() {
|
|
return this.replicationMode === 'dr' ? 'Disaster Recovery' : 'Performance';
|
|
}),
|
|
replicationIsInitializing: computed('dr.mode', 'performance.mode', function() {
|
|
// a mode of null only happens when a cluster is being initialized
|
|
// otherwise the mode will be 'disabled', 'primary', 'secondary'
|
|
return !this.dr.mode || !this.performance.mode;
|
|
}),
|
|
replicationAttrs: computed('dr.mode', 'performance.mode', 'replicationMode', function() {
|
|
const replicationMode = this.get('replicationMode');
|
|
return replicationMode ? get(this, replicationMode) : null;
|
|
}),
|
|
});
|