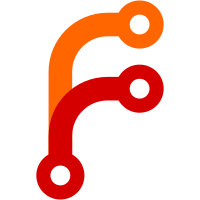
* agent: return a non-zero exit code on error * agent/template: always return on template server error, add case for error_on_missing_key * agent: fix tests by updating Run params to use an errCh * agent/template: add permission denied test case, clean up test var * agent: use unbuffered errCh, emit fatal errors directly to the UI output * agent: use oklog's run.Group to schedule subsystem runners (#9761) * agent: use oklog's run.Group to schedule subsystem runners * agent: clean up unused DoneCh, clean up agent's main Run func * agent/template: use ts.stopped.CAS to atomically swap value * fix tests * fix tests * agent/template: add timeout on TestRunServer * agent: output error via logs and return a generic error on non-zero exit * fix TestAgent_ExitAfterAuth * agent/template: do not restart ct runner on new incoming token if exit_after_auth is set to true * agent: drain ah.OutputCh after sink exits to avoid blocking on the channel * use context.WithTimeout, expand comments around ordering of defer cancel()
109 lines
2.4 KiB
Go
109 lines
2.4 KiB
Go
package auth
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
"testing"
|
|
"time"
|
|
|
|
hclog "github.com/hashicorp/go-hclog"
|
|
"github.com/hashicorp/vault/api"
|
|
"github.com/hashicorp/vault/builtin/credential/userpass"
|
|
vaulthttp "github.com/hashicorp/vault/http"
|
|
"github.com/hashicorp/vault/sdk/helper/logging"
|
|
"github.com/hashicorp/vault/sdk/logical"
|
|
"github.com/hashicorp/vault/vault"
|
|
)
|
|
|
|
type userpassTestMethod struct{}
|
|
|
|
func newUserpassTestMethod(t *testing.T, client *api.Client) AuthMethod {
|
|
err := client.Sys().EnableAuthWithOptions("userpass", &api.EnableAuthOptions{
|
|
Type: "userpass",
|
|
Config: api.AuthConfigInput{
|
|
DefaultLeaseTTL: "1s",
|
|
MaxLeaseTTL: "3s",
|
|
},
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
return &userpassTestMethod{}
|
|
}
|
|
|
|
func (u *userpassTestMethod) Authenticate(_ context.Context, client *api.Client) (string, http.Header, map[string]interface{}, error) {
|
|
_, err := client.Logical().Write("auth/userpass/users/foo", map[string]interface{}{
|
|
"password": "bar",
|
|
})
|
|
if err != nil {
|
|
return "", nil, nil, err
|
|
}
|
|
return "auth/userpass/login/foo", nil, map[string]interface{}{
|
|
"password": "bar",
|
|
}, nil
|
|
}
|
|
|
|
func (u *userpassTestMethod) NewCreds() chan struct{} {
|
|
return nil
|
|
}
|
|
|
|
func (u *userpassTestMethod) CredSuccess() {
|
|
}
|
|
|
|
func (u *userpassTestMethod) Shutdown() {
|
|
}
|
|
|
|
func TestAuthHandler(t *testing.T) {
|
|
logger := logging.NewVaultLogger(hclog.Trace)
|
|
coreConfig := &vault.CoreConfig{
|
|
Logger: logger,
|
|
CredentialBackends: map[string]logical.Factory{
|
|
"userpass": userpass.Factory,
|
|
},
|
|
}
|
|
cluster := vault.NewTestCluster(t, coreConfig, &vault.TestClusterOptions{
|
|
HandlerFunc: vaulthttp.Handler,
|
|
})
|
|
cluster.Start()
|
|
defer cluster.Cleanup()
|
|
|
|
vault.TestWaitActive(t, cluster.Cores[0].Core)
|
|
client := cluster.Cores[0].Client
|
|
|
|
ctx, cancelFunc := context.WithCancel(context.Background())
|
|
|
|
ah := NewAuthHandler(&AuthHandlerConfig{
|
|
Logger: logger.Named("auth.handler"),
|
|
Client: client,
|
|
})
|
|
|
|
am := newUserpassTestMethod(t, client)
|
|
errCh := make(chan error)
|
|
go func() {
|
|
errCh <- ah.Run(ctx, am)
|
|
}()
|
|
|
|
// Consume tokens so we don't block
|
|
stopTime := time.Now().Add(5 * time.Second)
|
|
closed := false
|
|
consumption:
|
|
for {
|
|
select {
|
|
case err := <-errCh:
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
break consumption
|
|
case <-ah.OutputCh:
|
|
case <-ah.TemplateTokenCh:
|
|
// Nothing
|
|
case <-time.After(stopTime.Sub(time.Now())):
|
|
if !closed {
|
|
cancelFunc()
|
|
closed = true
|
|
}
|
|
}
|
|
}
|
|
}
|