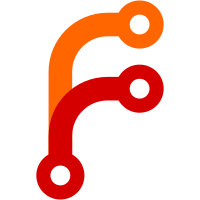
Move version out of SDK. For now it's a copy rather than move: the part not addressed by this change is sdk/helper/useragent.String, which we'll want to remove in favour of PluginString. That will have to wait until we've removed uses of useragent.String from all builtins.
80 lines
1.7 KiB
Go
80 lines
1.7 KiB
Go
package version
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
)
|
|
|
|
type VersionInfo struct {
|
|
Revision string `json:"revision,omitempty"`
|
|
Version string `json:"version,omitempty"`
|
|
VersionPrerelease string `json:"version_prerelease,omitempty"`
|
|
VersionMetadata string `json:"version_metadata,omitempty"`
|
|
BuildDate string `json:"build_date,omitempty"`
|
|
}
|
|
|
|
func GetVersion() *VersionInfo {
|
|
ver := Version
|
|
rel := VersionPrerelease
|
|
md := VersionMetadata
|
|
if GitDescribe != "" {
|
|
ver = GitDescribe
|
|
}
|
|
if GitDescribe == "" && rel == "" && VersionPrerelease != "" {
|
|
rel = "dev"
|
|
}
|
|
|
|
return &VersionInfo{
|
|
Revision: GitCommit,
|
|
Version: ver,
|
|
VersionPrerelease: rel,
|
|
VersionMetadata: md,
|
|
BuildDate: BuildDate,
|
|
}
|
|
}
|
|
|
|
func (c *VersionInfo) VersionNumber() string {
|
|
if Version == "unknown" && VersionPrerelease == "unknown" {
|
|
return "(version unknown)"
|
|
}
|
|
|
|
version := c.Version
|
|
|
|
if c.VersionPrerelease != "" {
|
|
version = fmt.Sprintf("%s-%s", version, c.VersionPrerelease)
|
|
}
|
|
|
|
if c.VersionMetadata != "" {
|
|
version = fmt.Sprintf("%s+%s", version, c.VersionMetadata)
|
|
}
|
|
|
|
return version
|
|
}
|
|
|
|
func (c *VersionInfo) FullVersionNumber(rev bool) string {
|
|
var versionString bytes.Buffer
|
|
|
|
if Version == "unknown" && VersionPrerelease == "unknown" {
|
|
return "Vault (version unknown)"
|
|
}
|
|
|
|
fmt.Fprintf(&versionString, "Vault v%s", c.Version)
|
|
if c.VersionPrerelease != "" {
|
|
fmt.Fprintf(&versionString, "-%s", c.VersionPrerelease)
|
|
}
|
|
|
|
if c.VersionMetadata != "" {
|
|
fmt.Fprintf(&versionString, "+%s", c.VersionMetadata)
|
|
}
|
|
|
|
if rev && c.Revision != "" {
|
|
fmt.Fprintf(&versionString, " (%s)", c.Revision)
|
|
}
|
|
|
|
if c.BuildDate != "" {
|
|
fmt.Fprintf(&versionString, ", built %s", c.BuildDate)
|
|
}
|
|
|
|
return versionString.String()
|
|
}
|