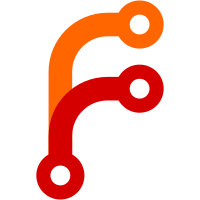
A lot of places took a (context, backend, request) tuple, ignoring the request proper and only using it for its storage. This (modified) tuple is exactly the set of elements in the shared storage context, so we should be using that instead of manually passing all three elements around. This simplifies a few places where we'd generate a storage context at the request level and then split it apart only to recreate it again later (e.g., CRL building). Signed-off-by: Alexander Scheel <alex.scheel@hashicorp.com> Signed-off-by: Alexander Scheel <alex.scheel@hashicorp.com>
58 lines
1.5 KiB
Go
58 lines
1.5 KiB
Go
package pki
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/hashicorp/vault/sdk/framework"
|
|
"github.com/hashicorp/vault/sdk/logical"
|
|
)
|
|
|
|
// SecretCertsType is the name used to identify this type
|
|
const SecretCertsType = "pki"
|
|
|
|
func secretCerts(b *backend) *framework.Secret {
|
|
return &framework.Secret{
|
|
Type: SecretCertsType,
|
|
Fields: map[string]*framework.FieldSchema{
|
|
"certificate": {
|
|
Type: framework.TypeString,
|
|
Description: `The PEM-encoded concatenated certificate and
|
|
issuing certificate authority`,
|
|
},
|
|
"private_key": {
|
|
Type: framework.TypeString,
|
|
Description: "The PEM-encoded private key for the certificate",
|
|
},
|
|
"serial": {
|
|
Type: framework.TypeString,
|
|
Description: `The serial number of the certificate, for handy
|
|
reference`,
|
|
},
|
|
},
|
|
|
|
Revoke: b.secretCredsRevoke,
|
|
}
|
|
}
|
|
|
|
func (b *backend) secretCredsRevoke(ctx context.Context, req *logical.Request, _ *framework.FieldData) (*logical.Response, error) {
|
|
if req.Secret == nil {
|
|
return nil, fmt.Errorf("secret is nil in request")
|
|
}
|
|
|
|
serialInt, ok := req.Secret.InternalData["serial_number"]
|
|
if !ok {
|
|
return nil, fmt.Errorf("could not find serial in internal secret data")
|
|
}
|
|
|
|
b.revokeStorageLock.Lock()
|
|
defer b.revokeStorageLock.Unlock()
|
|
|
|
sc := b.makeStorageContext(ctx, req.Storage)
|
|
resp, err := revokeCert(sc, serialInt.(string), true)
|
|
if resp == nil && err == nil {
|
|
b.Logger().Warn("expired certificate revoke failed because not found in storage, treating as success", "serial", serialInt.(string))
|
|
}
|
|
return resp, err
|
|
}
|