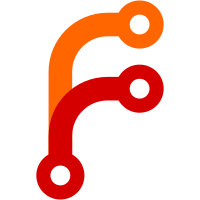
* Update browserslist * Add browserslistrc * ember-cli-update --to 3.26, fix conflicts * Run codemodes that start with ember-* * More codemods - before cp* * More codemods (curly data-test-*) * WIP ember-basic-dropdown template errors * updates ember-basic-dropdown and related deps to fix build issues * updates basic dropdown instances to new version API * updates more deps -- ember-template-lint is working again * runs no-implicit-this codemod * creates and runs no-quoteless-attributes codemod * runs angle brackets codemod * updates lint:hbs globs to only touch hbs files * removes yield only templates * creates and runs deprecated args transform * supresses lint error for invokeAction on LinkTo component * resolves remaining ambiguous path lint errors * resolves simple-unless lint errors * adds warnings for deprecated tagName arg on LinkTo components * adds warnings for remaining curly component invocation * updates global template lint rules * resolves remaining template lint errors * disables some ember specfic lint rules that target pre octane patterns * js lint fix run * resolves remaining js lint errors * fixes test run * adds npm-run-all dep * fixes test attribute issues * fixes console acceptance tests * fixes tests * adds yield only wizard/tutorial-active template * fixes more tests * attempts to fix more flaky tests * removes commented out settled in transit test * updates deprecations workflow and adds initializer to filter by version * updates flaky policies acl old test * updates to flaky transit test * bumps ember deps down to LTS version * runs linters after main merge * fixes client count tests after bad merge conflict fixes * fixes client count history test * more updates to lint config * another round of hbs lint fixes after extending stylistic rule * updates lint-staged commands * removes indent eslint rule since it seems to break things * fixes bad attribute in transform-edit-form template * test fixes * fixes enterprise tests * adds changelog * removes deprecated ember-concurrency-test-waiters dep and adds @ember/test-waiters * flaky test fix Co-authored-by: hashishaw <cshaw@hashicorp.com>
125 lines
3.9 KiB
JavaScript
125 lines
3.9 KiB
JavaScript
import Component from '@glimmer/component';
|
|
import { action } from '@ember/object';
|
|
import { tracked } from '@glimmer/tracking';
|
|
import { format } from 'date-fns';
|
|
|
|
export default class HistoryComponent extends Component {
|
|
max_namespaces = 10;
|
|
|
|
@tracked selectedNamespace = null;
|
|
|
|
@tracked barChartSelection = false;
|
|
|
|
// Determine if we have client count data based on the current tab
|
|
get hasClientData() {
|
|
if (this.args.tab === 'current') {
|
|
// Show the current numbers as long as config is on
|
|
return this.args.model.config?.enabled !== 'Off';
|
|
}
|
|
return this.args.model.activity && this.args.model.activity.total;
|
|
}
|
|
|
|
// Show namespace graph only if we have more than 1
|
|
get showGraphs() {
|
|
return (
|
|
this.args.model.activity &&
|
|
this.args.model.activity.byNamespace &&
|
|
this.args.model.activity.byNamespace.length > 1
|
|
);
|
|
}
|
|
|
|
// Construct the namespace model for the search select component
|
|
get searchDataset() {
|
|
if (!this.args.model.activity || !this.args.model.activity.byNamespace) {
|
|
return null;
|
|
}
|
|
let dataList = this.args.model.activity.byNamespace;
|
|
return dataList.map((d) => {
|
|
return {
|
|
name: d['namespace_id'],
|
|
id: d['namespace_path'] === '' ? 'root' : d['namespace_path'],
|
|
};
|
|
});
|
|
}
|
|
|
|
// Construct the namespace model for the bar chart component
|
|
get barChartDataset() {
|
|
if (!this.args.model.activity || !this.args.model.activity.byNamespace) {
|
|
return null;
|
|
}
|
|
let dataset = this.args.model.activity.byNamespace.slice(0, this.max_namespaces);
|
|
return dataset.map((d) => {
|
|
return {
|
|
label: d['namespace_path'] === '' ? 'root' : d['namespace_path'],
|
|
non_entity_tokens: d['counts']['non_entity_tokens'],
|
|
distinct_entities: d['counts']['distinct_entities'],
|
|
total: d['counts']['clients'],
|
|
};
|
|
});
|
|
}
|
|
|
|
// Create namespaces data for csv format
|
|
get getCsvData() {
|
|
if (!this.args.model.activity || !this.args.model.activity.byNamespace) {
|
|
return null;
|
|
}
|
|
let results = '',
|
|
namespaces = this.args.model.activity.byNamespace,
|
|
fields = ['Namespace path', 'Active clients', 'Unique entities', 'Non-entity tokens'];
|
|
|
|
results = fields.join(',') + '\n';
|
|
|
|
namespaces.forEach(function (item) {
|
|
let path = item.namespace_path !== '' ? item.namespace_path : 'root',
|
|
total = item.counts.clients,
|
|
unique = item.counts.distinct_entities,
|
|
non_entity = item.counts.non_entity_tokens;
|
|
|
|
results += path + ',' + total + ',' + unique + ',' + non_entity + '\n';
|
|
});
|
|
return results;
|
|
}
|
|
|
|
// Return csv filename with start and end dates
|
|
get getCsvFileName() {
|
|
let defaultFileName = `clients-by-namespace`,
|
|
startDate =
|
|
this.args.model.queryStart || `${format(new Date(this.args.model.activity.startTime), 'MM-yyyy')}`,
|
|
endDate =
|
|
this.args.model.queryEnd || `${format(new Date(this.args.model.activity.endTime), 'MM-yyyy')}`;
|
|
if (startDate && endDate) {
|
|
defaultFileName += `-${startDate}-${endDate}`;
|
|
}
|
|
return defaultFileName;
|
|
}
|
|
|
|
// Get the namespace by matching the path from the namespace list
|
|
getNamespace(path) {
|
|
return this.args.model.activity.byNamespace.find((ns) => {
|
|
if (path === 'root') {
|
|
return ns.namespace_path === '';
|
|
}
|
|
return ns.namespace_path === path;
|
|
});
|
|
}
|
|
|
|
@action
|
|
selectNamespace(value) {
|
|
// In case of search select component, value returned is an array
|
|
if (Array.isArray(value)) {
|
|
this.selectedNamespace = this.getNamespace(value[0]);
|
|
this.barChartSelection = false;
|
|
} else if (typeof value === 'object') {
|
|
// While D3 bar selection returns an object
|
|
this.selectedNamespace = this.getNamespace(value.label);
|
|
this.barChartSelection = true;
|
|
}
|
|
}
|
|
|
|
@action
|
|
resetData() {
|
|
this.barChartSelection = false;
|
|
this.selectedNamespace = null;
|
|
}
|
|
}
|