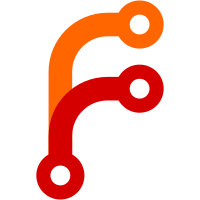
* Start work on context aware backends * Start work on moving the database plugins to gRPC in order to pass context * Add context to builtin database plugins * use byte slice instead of string * Context all the things * Move proto messages to the dbplugin package * Add a grpc mechanism for running backend plugins * Serve the GRPC plugin * Add backwards compatibility to the database plugins * Remove backend plugin changes * Remove backend plugin changes * Cleanup the transport implementations * If grpc connection is in an unexpected state restart the plugin * Fix tests * Fix tests * Remove context from the request object, replace it with context.TODO * Add a test to verify netRPC plugins still work * Remove unused mapstructure call * Code review fixes * Code review fixes * Code review fixes
557 lines
20 KiB
Go
557 lines
20 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// source: builtin/logical/database/dbplugin/database.proto
|
|
|
|
/*
|
|
Package dbplugin is a generated protocol buffer package.
|
|
|
|
It is generated from these files:
|
|
builtin/logical/database/dbplugin/database.proto
|
|
|
|
It has these top-level messages:
|
|
InitializeRequest
|
|
CreateUserRequest
|
|
RenewUserRequest
|
|
RevokeUserRequest
|
|
Statements
|
|
UsernameConfig
|
|
CreateUserResponse
|
|
TypeResponse
|
|
Empty
|
|
*/
|
|
package dbplugin
|
|
|
|
import proto "github.com/golang/protobuf/proto"
|
|
import fmt "fmt"
|
|
import math "math"
|
|
import google_protobuf "github.com/golang/protobuf/ptypes/timestamp"
|
|
|
|
import (
|
|
context "golang.org/x/net/context"
|
|
grpc "google.golang.org/grpc"
|
|
)
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the proto package it is being compiled against.
|
|
// A compilation error at this line likely means your copy of the
|
|
// proto package needs to be updated.
|
|
const _ = proto.ProtoPackageIsVersion2 // please upgrade the proto package
|
|
|
|
type InitializeRequest struct {
|
|
Config []byte `protobuf:"bytes,1,opt,name=config,proto3" json:"config,omitempty"`
|
|
VerifyConnection bool `protobuf:"varint,2,opt,name=verify_connection,json=verifyConnection" json:"verify_connection,omitempty"`
|
|
}
|
|
|
|
func (m *InitializeRequest) Reset() { *m = InitializeRequest{} }
|
|
func (m *InitializeRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*InitializeRequest) ProtoMessage() {}
|
|
func (*InitializeRequest) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{0} }
|
|
|
|
func (m *InitializeRequest) GetConfig() []byte {
|
|
if m != nil {
|
|
return m.Config
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *InitializeRequest) GetVerifyConnection() bool {
|
|
if m != nil {
|
|
return m.VerifyConnection
|
|
}
|
|
return false
|
|
}
|
|
|
|
type CreateUserRequest struct {
|
|
Statements *Statements `protobuf:"bytes,1,opt,name=statements" json:"statements,omitempty"`
|
|
UsernameConfig *UsernameConfig `protobuf:"bytes,2,opt,name=username_config,json=usernameConfig" json:"username_config,omitempty"`
|
|
Expiration *google_protobuf.Timestamp `protobuf:"bytes,3,opt,name=expiration" json:"expiration,omitempty"`
|
|
}
|
|
|
|
func (m *CreateUserRequest) Reset() { *m = CreateUserRequest{} }
|
|
func (m *CreateUserRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*CreateUserRequest) ProtoMessage() {}
|
|
func (*CreateUserRequest) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{1} }
|
|
|
|
func (m *CreateUserRequest) GetStatements() *Statements {
|
|
if m != nil {
|
|
return m.Statements
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *CreateUserRequest) GetUsernameConfig() *UsernameConfig {
|
|
if m != nil {
|
|
return m.UsernameConfig
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *CreateUserRequest) GetExpiration() *google_protobuf.Timestamp {
|
|
if m != nil {
|
|
return m.Expiration
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RenewUserRequest struct {
|
|
Statements *Statements `protobuf:"bytes,1,opt,name=statements" json:"statements,omitempty"`
|
|
Username string `protobuf:"bytes,2,opt,name=username" json:"username,omitempty"`
|
|
Expiration *google_protobuf.Timestamp `protobuf:"bytes,3,opt,name=expiration" json:"expiration,omitempty"`
|
|
}
|
|
|
|
func (m *RenewUserRequest) Reset() { *m = RenewUserRequest{} }
|
|
func (m *RenewUserRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*RenewUserRequest) ProtoMessage() {}
|
|
func (*RenewUserRequest) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{2} }
|
|
|
|
func (m *RenewUserRequest) GetStatements() *Statements {
|
|
if m != nil {
|
|
return m.Statements
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *RenewUserRequest) GetUsername() string {
|
|
if m != nil {
|
|
return m.Username
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *RenewUserRequest) GetExpiration() *google_protobuf.Timestamp {
|
|
if m != nil {
|
|
return m.Expiration
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type RevokeUserRequest struct {
|
|
Statements *Statements `protobuf:"bytes,1,opt,name=statements" json:"statements,omitempty"`
|
|
Username string `protobuf:"bytes,2,opt,name=username" json:"username,omitempty"`
|
|
}
|
|
|
|
func (m *RevokeUserRequest) Reset() { *m = RevokeUserRequest{} }
|
|
func (m *RevokeUserRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*RevokeUserRequest) ProtoMessage() {}
|
|
func (*RevokeUserRequest) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{3} }
|
|
|
|
func (m *RevokeUserRequest) GetStatements() *Statements {
|
|
if m != nil {
|
|
return m.Statements
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (m *RevokeUserRequest) GetUsername() string {
|
|
if m != nil {
|
|
return m.Username
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type Statements struct {
|
|
CreationStatements string `protobuf:"bytes,1,opt,name=creation_statements,json=creationStatements" json:"creation_statements,omitempty"`
|
|
RevocationStatements string `protobuf:"bytes,2,opt,name=revocation_statements,json=revocationStatements" json:"revocation_statements,omitempty"`
|
|
RollbackStatements string `protobuf:"bytes,3,opt,name=rollback_statements,json=rollbackStatements" json:"rollback_statements,omitempty"`
|
|
RenewStatements string `protobuf:"bytes,4,opt,name=renew_statements,json=renewStatements" json:"renew_statements,omitempty"`
|
|
}
|
|
|
|
func (m *Statements) Reset() { *m = Statements{} }
|
|
func (m *Statements) String() string { return proto.CompactTextString(m) }
|
|
func (*Statements) ProtoMessage() {}
|
|
func (*Statements) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{4} }
|
|
|
|
func (m *Statements) GetCreationStatements() string {
|
|
if m != nil {
|
|
return m.CreationStatements
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *Statements) GetRevocationStatements() string {
|
|
if m != nil {
|
|
return m.RevocationStatements
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *Statements) GetRollbackStatements() string {
|
|
if m != nil {
|
|
return m.RollbackStatements
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *Statements) GetRenewStatements() string {
|
|
if m != nil {
|
|
return m.RenewStatements
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type UsernameConfig struct {
|
|
DisplayName string `protobuf:"bytes,1,opt,name=DisplayName" json:"DisplayName,omitempty"`
|
|
RoleName string `protobuf:"bytes,2,opt,name=RoleName" json:"RoleName,omitempty"`
|
|
}
|
|
|
|
func (m *UsernameConfig) Reset() { *m = UsernameConfig{} }
|
|
func (m *UsernameConfig) String() string { return proto.CompactTextString(m) }
|
|
func (*UsernameConfig) ProtoMessage() {}
|
|
func (*UsernameConfig) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{5} }
|
|
|
|
func (m *UsernameConfig) GetDisplayName() string {
|
|
if m != nil {
|
|
return m.DisplayName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *UsernameConfig) GetRoleName() string {
|
|
if m != nil {
|
|
return m.RoleName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type CreateUserResponse struct {
|
|
Username string `protobuf:"bytes,1,opt,name=username" json:"username,omitempty"`
|
|
Password string `protobuf:"bytes,2,opt,name=password" json:"password,omitempty"`
|
|
}
|
|
|
|
func (m *CreateUserResponse) Reset() { *m = CreateUserResponse{} }
|
|
func (m *CreateUserResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*CreateUserResponse) ProtoMessage() {}
|
|
func (*CreateUserResponse) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{6} }
|
|
|
|
func (m *CreateUserResponse) GetUsername() string {
|
|
if m != nil {
|
|
return m.Username
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (m *CreateUserResponse) GetPassword() string {
|
|
if m != nil {
|
|
return m.Password
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type TypeResponse struct {
|
|
Type string `protobuf:"bytes,1,opt,name=type" json:"type,omitempty"`
|
|
}
|
|
|
|
func (m *TypeResponse) Reset() { *m = TypeResponse{} }
|
|
func (m *TypeResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*TypeResponse) ProtoMessage() {}
|
|
func (*TypeResponse) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{7} }
|
|
|
|
func (m *TypeResponse) GetType() string {
|
|
if m != nil {
|
|
return m.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type Empty struct {
|
|
}
|
|
|
|
func (m *Empty) Reset() { *m = Empty{} }
|
|
func (m *Empty) String() string { return proto.CompactTextString(m) }
|
|
func (*Empty) ProtoMessage() {}
|
|
func (*Empty) Descriptor() ([]byte, []int) { return fileDescriptor0, []int{8} }
|
|
|
|
func init() {
|
|
proto.RegisterType((*InitializeRequest)(nil), "dbplugin.InitializeRequest")
|
|
proto.RegisterType((*CreateUserRequest)(nil), "dbplugin.CreateUserRequest")
|
|
proto.RegisterType((*RenewUserRequest)(nil), "dbplugin.RenewUserRequest")
|
|
proto.RegisterType((*RevokeUserRequest)(nil), "dbplugin.RevokeUserRequest")
|
|
proto.RegisterType((*Statements)(nil), "dbplugin.Statements")
|
|
proto.RegisterType((*UsernameConfig)(nil), "dbplugin.UsernameConfig")
|
|
proto.RegisterType((*CreateUserResponse)(nil), "dbplugin.CreateUserResponse")
|
|
proto.RegisterType((*TypeResponse)(nil), "dbplugin.TypeResponse")
|
|
proto.RegisterType((*Empty)(nil), "dbplugin.Empty")
|
|
}
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ context.Context
|
|
var _ grpc.ClientConn
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the grpc package it is being compiled against.
|
|
const _ = grpc.SupportPackageIsVersion4
|
|
|
|
// Client API for Database service
|
|
|
|
type DatabaseClient interface {
|
|
Type(ctx context.Context, in *Empty, opts ...grpc.CallOption) (*TypeResponse, error)
|
|
CreateUser(ctx context.Context, in *CreateUserRequest, opts ...grpc.CallOption) (*CreateUserResponse, error)
|
|
RenewUser(ctx context.Context, in *RenewUserRequest, opts ...grpc.CallOption) (*Empty, error)
|
|
RevokeUser(ctx context.Context, in *RevokeUserRequest, opts ...grpc.CallOption) (*Empty, error)
|
|
Initialize(ctx context.Context, in *InitializeRequest, opts ...grpc.CallOption) (*Empty, error)
|
|
Close(ctx context.Context, in *Empty, opts ...grpc.CallOption) (*Empty, error)
|
|
}
|
|
|
|
type databaseClient struct {
|
|
cc *grpc.ClientConn
|
|
}
|
|
|
|
func NewDatabaseClient(cc *grpc.ClientConn) DatabaseClient {
|
|
return &databaseClient{cc}
|
|
}
|
|
|
|
func (c *databaseClient) Type(ctx context.Context, in *Empty, opts ...grpc.CallOption) (*TypeResponse, error) {
|
|
out := new(TypeResponse)
|
|
err := grpc.Invoke(ctx, "/dbplugin.Database/Type", in, out, c.cc, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *databaseClient) CreateUser(ctx context.Context, in *CreateUserRequest, opts ...grpc.CallOption) (*CreateUserResponse, error) {
|
|
out := new(CreateUserResponse)
|
|
err := grpc.Invoke(ctx, "/dbplugin.Database/CreateUser", in, out, c.cc, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *databaseClient) RenewUser(ctx context.Context, in *RenewUserRequest, opts ...grpc.CallOption) (*Empty, error) {
|
|
out := new(Empty)
|
|
err := grpc.Invoke(ctx, "/dbplugin.Database/RenewUser", in, out, c.cc, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *databaseClient) RevokeUser(ctx context.Context, in *RevokeUserRequest, opts ...grpc.CallOption) (*Empty, error) {
|
|
out := new(Empty)
|
|
err := grpc.Invoke(ctx, "/dbplugin.Database/RevokeUser", in, out, c.cc, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *databaseClient) Initialize(ctx context.Context, in *InitializeRequest, opts ...grpc.CallOption) (*Empty, error) {
|
|
out := new(Empty)
|
|
err := grpc.Invoke(ctx, "/dbplugin.Database/Initialize", in, out, c.cc, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
func (c *databaseClient) Close(ctx context.Context, in *Empty, opts ...grpc.CallOption) (*Empty, error) {
|
|
out := new(Empty)
|
|
err := grpc.Invoke(ctx, "/dbplugin.Database/Close", in, out, c.cc, opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return out, nil
|
|
}
|
|
|
|
// Server API for Database service
|
|
|
|
type DatabaseServer interface {
|
|
Type(context.Context, *Empty) (*TypeResponse, error)
|
|
CreateUser(context.Context, *CreateUserRequest) (*CreateUserResponse, error)
|
|
RenewUser(context.Context, *RenewUserRequest) (*Empty, error)
|
|
RevokeUser(context.Context, *RevokeUserRequest) (*Empty, error)
|
|
Initialize(context.Context, *InitializeRequest) (*Empty, error)
|
|
Close(context.Context, *Empty) (*Empty, error)
|
|
}
|
|
|
|
func RegisterDatabaseServer(s *grpc.Server, srv DatabaseServer) {
|
|
s.RegisterService(&_Database_serviceDesc, srv)
|
|
}
|
|
|
|
func _Database_Type_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(DatabaseServer).Type(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/dbplugin.Database/Type",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(DatabaseServer).Type(ctx, req.(*Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _Database_CreateUser_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(CreateUserRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(DatabaseServer).CreateUser(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/dbplugin.Database/CreateUser",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(DatabaseServer).CreateUser(ctx, req.(*CreateUserRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _Database_RenewUser_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(RenewUserRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(DatabaseServer).RenewUser(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/dbplugin.Database/RenewUser",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(DatabaseServer).RenewUser(ctx, req.(*RenewUserRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _Database_RevokeUser_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(RevokeUserRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(DatabaseServer).RevokeUser(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/dbplugin.Database/RevokeUser",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(DatabaseServer).RevokeUser(ctx, req.(*RevokeUserRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _Database_Initialize_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(InitializeRequest)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(DatabaseServer).Initialize(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/dbplugin.Database/Initialize",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(DatabaseServer).Initialize(ctx, req.(*InitializeRequest))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
func _Database_Close_Handler(srv interface{}, ctx context.Context, dec func(interface{}) error, interceptor grpc.UnaryServerInterceptor) (interface{}, error) {
|
|
in := new(Empty)
|
|
if err := dec(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if interceptor == nil {
|
|
return srv.(DatabaseServer).Close(ctx, in)
|
|
}
|
|
info := &grpc.UnaryServerInfo{
|
|
Server: srv,
|
|
FullMethod: "/dbplugin.Database/Close",
|
|
}
|
|
handler := func(ctx context.Context, req interface{}) (interface{}, error) {
|
|
return srv.(DatabaseServer).Close(ctx, req.(*Empty))
|
|
}
|
|
return interceptor(ctx, in, info, handler)
|
|
}
|
|
|
|
var _Database_serviceDesc = grpc.ServiceDesc{
|
|
ServiceName: "dbplugin.Database",
|
|
HandlerType: (*DatabaseServer)(nil),
|
|
Methods: []grpc.MethodDesc{
|
|
{
|
|
MethodName: "Type",
|
|
Handler: _Database_Type_Handler,
|
|
},
|
|
{
|
|
MethodName: "CreateUser",
|
|
Handler: _Database_CreateUser_Handler,
|
|
},
|
|
{
|
|
MethodName: "RenewUser",
|
|
Handler: _Database_RenewUser_Handler,
|
|
},
|
|
{
|
|
MethodName: "RevokeUser",
|
|
Handler: _Database_RevokeUser_Handler,
|
|
},
|
|
{
|
|
MethodName: "Initialize",
|
|
Handler: _Database_Initialize_Handler,
|
|
},
|
|
{
|
|
MethodName: "Close",
|
|
Handler: _Database_Close_Handler,
|
|
},
|
|
},
|
|
Streams: []grpc.StreamDesc{},
|
|
Metadata: "builtin/logical/database/dbplugin/database.proto",
|
|
}
|
|
|
|
func init() { proto.RegisterFile("builtin/logical/database/dbplugin/database.proto", fileDescriptor0) }
|
|
|
|
var fileDescriptor0 = []byte{
|
|
// 548 bytes of a gzipped FileDescriptorProto
|
|
0x1f, 0x8b, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0xff, 0xb4, 0x54, 0xcf, 0x6e, 0xd3, 0x4e,
|
|
0x10, 0x96, 0xdb, 0xb4, 0xbf, 0x64, 0x5a, 0x35, 0xc9, 0xfe, 0x4a, 0x15, 0x19, 0x24, 0x22, 0x9f,
|
|
0x5a, 0x21, 0xd9, 0xa8, 0xe5, 0x80, 0xb8, 0xa1, 0x14, 0x21, 0x24, 0x94, 0x83, 0x69, 0x25, 0x6e,
|
|
0xd1, 0xda, 0x99, 0x44, 0xab, 0x3a, 0xbb, 0xc6, 0xbb, 0x4e, 0x09, 0x4f, 0xc3, 0xe3, 0x70, 0xe2,
|
|
0x1d, 0x78, 0x13, 0xe4, 0x75, 0xd6, 0xbb, 0xf9, 0x73, 0xab, 0xb8, 0x79, 0x66, 0xbe, 0x6f, 0xf6,
|
|
0xf3, 0xb7, 0x33, 0x0b, 0xaf, 0x93, 0x92, 0x65, 0x8a, 0xf1, 0x28, 0x13, 0x73, 0x96, 0xd2, 0x2c,
|
|
0x9a, 0x52, 0x45, 0x13, 0x2a, 0x31, 0x9a, 0x26, 0x79, 0x56, 0xce, 0x19, 0x6f, 0x32, 0x61, 0x5e,
|
|
0x08, 0x25, 0x48, 0xdb, 0x14, 0xfc, 0x97, 0x73, 0x21, 0xe6, 0x19, 0x46, 0x3a, 0x9f, 0x94, 0xb3,
|
|
0x48, 0xb1, 0x05, 0x4a, 0x45, 0x17, 0x79, 0x0d, 0x0d, 0xbe, 0x42, 0xff, 0x13, 0x67, 0x8a, 0xd1,
|
|
0x8c, 0xfd, 0xc0, 0x18, 0xbf, 0x95, 0x28, 0x15, 0xb9, 0x80, 0xe3, 0x54, 0xf0, 0x19, 0x9b, 0x0f,
|
|
0xbc, 0xa1, 0x77, 0x79, 0x1a, 0xaf, 0x23, 0xf2, 0x0a, 0xfa, 0x4b, 0x2c, 0xd8, 0x6c, 0x35, 0x49,
|
|
0x05, 0xe7, 0x98, 0x2a, 0x26, 0xf8, 0xe0, 0x60, 0xe8, 0x5d, 0xb6, 0xe3, 0x5e, 0x5d, 0x18, 0x35,
|
|
0xf9, 0xe0, 0x97, 0x07, 0xfd, 0x51, 0x81, 0x54, 0xe1, 0xbd, 0xc4, 0xc2, 0xb4, 0x7e, 0x03, 0x20,
|
|
0x15, 0x55, 0xb8, 0x40, 0xae, 0xa4, 0x6e, 0x7f, 0x72, 0x7d, 0x1e, 0x1a, 0xbd, 0xe1, 0x97, 0xa6,
|
|
0x16, 0x3b, 0x38, 0xf2, 0x1e, 0xba, 0xa5, 0xc4, 0x82, 0xd3, 0x05, 0x4e, 0xd6, 0xca, 0x0e, 0x34,
|
|
0x75, 0x60, 0xa9, 0xf7, 0x6b, 0xc0, 0x48, 0xd7, 0xe3, 0xb3, 0x72, 0x23, 0x26, 0xef, 0x00, 0xf0,
|
|
0x7b, 0xce, 0x0a, 0xaa, 0x45, 0x1f, 0x6a, 0xb6, 0x1f, 0xd6, 0xf6, 0x84, 0xc6, 0x9e, 0xf0, 0xce,
|
|
0xd8, 0x13, 0x3b, 0xe8, 0xe0, 0xa7, 0x07, 0xbd, 0x18, 0x39, 0x3e, 0x3e, 0xfd, 0x4f, 0x7c, 0x68,
|
|
0x1b, 0x61, 0xfa, 0x17, 0x3a, 0x71, 0x13, 0x3f, 0x49, 0x22, 0x42, 0x3f, 0xc6, 0xa5, 0x78, 0xc0,
|
|
0x7f, 0x2a, 0x31, 0xf8, 0xed, 0x01, 0x58, 0x1a, 0x89, 0xe0, 0xff, 0xb4, 0xba, 0x62, 0x26, 0xf8,
|
|
0x64, 0xeb, 0xa4, 0x4e, 0x4c, 0x4c, 0xc9, 0x21, 0xdc, 0xc0, 0xb3, 0x02, 0x97, 0x22, 0xdd, 0xa1,
|
|
0xd4, 0x07, 0x9d, 0xdb, 0xe2, 0xe6, 0x29, 0x85, 0xc8, 0xb2, 0x84, 0xa6, 0x0f, 0x2e, 0xe5, 0xb0,
|
|
0x3e, 0xc5, 0x94, 0x1c, 0xc2, 0x15, 0xf4, 0x8a, 0xea, 0xba, 0x5c, 0x74, 0x4b, 0xa3, 0xbb, 0x3a,
|
|
0x6f, 0xa1, 0xc1, 0x18, 0xce, 0x36, 0x07, 0x87, 0x0c, 0xe1, 0xe4, 0x96, 0xc9, 0x3c, 0xa3, 0xab,
|
|
0x71, 0xe5, 0x40, 0xfd, 0x2f, 0x6e, 0xaa, 0x32, 0x28, 0x16, 0x19, 0x8e, 0x1d, 0x83, 0x4c, 0x1c,
|
|
0x7c, 0x06, 0xe2, 0x0e, 0xbd, 0xcc, 0x05, 0x97, 0xb8, 0x61, 0xa9, 0xb7, 0x75, 0xeb, 0x3e, 0xb4,
|
|
0x73, 0x2a, 0xe5, 0xa3, 0x28, 0xa6, 0xa6, 0x9b, 0x89, 0x83, 0x00, 0x4e, 0xef, 0x56, 0x39, 0x36,
|
|
0x7d, 0x08, 0xb4, 0xd4, 0x2a, 0x37, 0x3d, 0xf4, 0x77, 0xf0, 0x1f, 0x1c, 0x7d, 0x58, 0xe4, 0x6a,
|
|
0x75, 0xfd, 0xe7, 0x00, 0xda, 0xb7, 0xeb, 0x87, 0x80, 0x44, 0xd0, 0xaa, 0x98, 0xa4, 0x6b, 0xaf,
|
|
0x5b, 0xa3, 0xfc, 0x0b, 0x9b, 0xd8, 0x68, 0xfd, 0x11, 0xc0, 0x0a, 0x27, 0xcf, 0x2d, 0x6a, 0x67,
|
|
0x87, 0xfd, 0x17, 0xfb, 0x8b, 0xeb, 0x46, 0x6f, 0xa1, 0xd3, 0xec, 0x0a, 0xf1, 0x2d, 0x74, 0x7b,
|
|
0x81, 0xfc, 0x6d, 0x69, 0xd5, 0xfc, 0xdb, 0x19, 0x76, 0x25, 0xec, 0x4c, 0xf6, 0x5e, 0xae, 0x7d,
|
|
0xc7, 0x5c, 0xee, 0xce, 0xeb, 0xb6, 0xcb, 0xbd, 0x82, 0xa3, 0x51, 0x26, 0xe4, 0x1e, 0xb3, 0xb6,
|
|
0x13, 0xc9, 0xb1, 0x5e, 0xc3, 0x9b, 0xbf, 0x01, 0x00, 0x00, 0xff, 0xff, 0x8c, 0x55, 0x84, 0x56,
|
|
0x94, 0x05, 0x00, 0x00,
|
|
}
|