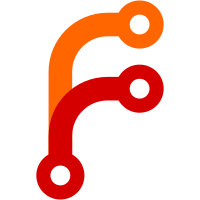
* Redo the API client quite a bit to make the behavior of NewClient more predictable and add locking to make it safer to use with Clone() and if multiple goroutines for some reason decide to change things. Along the way I discovered that currently, the x/net/http2 package is broke with the built-in h2 support in released Go. For those using DefaultConfig (the vast majority of cases) this will be a non-event. Others can manually call http2.ConfigureTransport as needed. We should keep an eye on commits on that repo and consider more updates before release. Alternately we could go back revisions but miss out on bug fixes; my theory is that this is not a purposeful break and I'll be following up on this in the Go issue tracker. In a few tests that don't use NewTestCluster, either for legacy or other reasons, ensure that http2.ConfigureTransport is called. * Use tls config cloning * Don't http2.ConfigureServer anymore as current Go seems to work properly without requiring the http2 package * Address feedback
27 lines
515 B
Go
27 lines
515 B
Go
package api
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"net/http"
|
|
"testing"
|
|
)
|
|
|
|
// testHTTPServer creates a test HTTP server that handles requests until
|
|
// the listener returned is closed.
|
|
func testHTTPServer(
|
|
t *testing.T, handler http.Handler) (*Config, net.Listener) {
|
|
ln, err := net.Listen("tcp", "127.0.0.1:0")
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
server := &http.Server{Handler: handler}
|
|
go server.Serve(ln)
|
|
|
|
config := DefaultConfig()
|
|
config.Address = fmt.Sprintf("http://%s", ln.Addr())
|
|
|
|
return config, ln
|
|
}
|