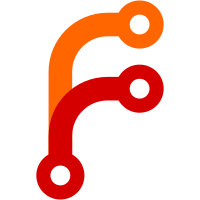
* adds lang attribute * fixes: empty anchor tag * adds alt attributes * alt tag logo grid updates * fix footer contrast color * only render header if it exists * adds `main` element to page * testing pre-releases * fix: button aria-label updates * chore: update deps * fix: adds `main` element to all pages * chore: formatting * fix: adds alts to use-cases page * chore: update headline element * chore: adds alt text * fix: adds alt tags * style: fix height issue * fix: use h1 at top of page * fix: remove main to avoid duplicate tag * chore: fix deps * main is already defined in docs page component * Update website/components/footer/style.css Co-authored-by: Jimmy Merritello <7191639+jmfury@users.noreply.github.com> Co-authored-by: Jimmy Merritello <7191639+jmfury@users.noreply.github.com>
65 lines
2.1 KiB
JavaScript
65 lines
2.1 KiB
JavaScript
import SectionHeader from '@hashicorp/react-section-header'
|
|
import Button from '@hashicorp/react-button'
|
|
import TextSplits from '@hashicorp/react-text-splits'
|
|
import BeforeAfterDiagram from 'components/before-after-diagram'
|
|
import UseCaseCtaSection from 'components/use-case-cta-section'
|
|
// Imports below are used in getStaticProps
|
|
import RAW_CONTENT from './content.json'
|
|
import highlightData from '@hashicorp/nextjs-scripts/prism/highlight-data'
|
|
import processBeforeAfterDiagramProps from 'components/before-after-diagram/server'
|
|
|
|
export async function getStaticProps() {
|
|
const content = await highlightData(RAW_CONTENT)
|
|
content.beforeAfterDiagram = await processBeforeAfterDiagramProps(
|
|
content.beforeAfterDiagram
|
|
)
|
|
return { props: { content } }
|
|
}
|
|
|
|
export default function DataEncryptionUseCase({ content }) {
|
|
return (
|
|
<main id="use-cases" className="g-section-block page-wrap">
|
|
{/* Header / Buttons */}
|
|
<section className="g-container">
|
|
<SectionHeader
|
|
headline="Encrypt Application Data in Low Trust Networks"
|
|
description="Keep application data secure with one centralized workflow to encrypt data in flight and at rest"
|
|
useH1={true}
|
|
/>
|
|
|
|
<div className="button-container">
|
|
<Button
|
|
title="Download"
|
|
label="Download CLI"
|
|
url="/downloads"
|
|
theme={{ brand: 'vault' }}
|
|
/>
|
|
<Button
|
|
title="Get Started"
|
|
label="Get started — external link to education platform"
|
|
url="/intro"
|
|
theme="dark-outline"
|
|
/>
|
|
</div>
|
|
</section>
|
|
|
|
{/* Before/After Diagram */}
|
|
<section>
|
|
<div className="g-container">
|
|
<BeforeAfterDiagram {...content.beforeAfterDiagram} />
|
|
</div>
|
|
</section>
|
|
|
|
{/* Features */}
|
|
<section className="no-section-spacing">
|
|
<div className="g-grid-container">
|
|
<SectionHeader headline=" Encryption Features" />
|
|
</div>
|
|
<TextSplits textSplits={content.features} />
|
|
</section>
|
|
|
|
<UseCaseCtaSection />
|
|
</main>
|
|
)
|
|
}
|