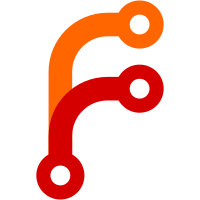
* vault-agent-cache: squashed 250+ commits * Add proper token revocation validations to the tests * Add more test cases * Avoid leaking by not closing request/response bodies; add comments * Fix revoke orphan use case; update tests * Add CLI test for making request over unix socket * agent/cache: remove namespace-related tests * Strip-off the auto-auth token from the lookup response * Output listener details along with configuration * Add scheme to API address output * leasecache: use IndexNameLease for prefix lease revocations * Make CLI accept the fully qualified unix address * export VAULT_AGENT_ADDR=unix://path/to/socket * unix:/ to unix://
37 lines
983 B
Go
37 lines
983 B
Go
package cache
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
)
|
|
|
|
// mockProxier is a mock implementation of the Proxier interface, used for testing purposes.
|
|
// The mock will return the provided responses every time it reaches its Send method, up to
|
|
// the last provided response. This lets tests control what the next/underlying Proxier layer
|
|
// might expect to return.
|
|
type mockProxier struct {
|
|
proxiedResponses []*SendResponse
|
|
responseIndex int
|
|
}
|
|
|
|
func newMockProxier(responses []*SendResponse) *mockProxier {
|
|
return &mockProxier{
|
|
proxiedResponses: responses,
|
|
}
|
|
}
|
|
|
|
func (p *mockProxier) Send(ctx context.Context, req *SendRequest) (*SendResponse, error) {
|
|
if p.responseIndex >= len(p.proxiedResponses) {
|
|
return nil, fmt.Errorf("index out of bounds: responseIndex = %d, responses = %d", p.responseIndex, len(p.proxiedResponses))
|
|
}
|
|
resp := p.proxiedResponses[p.responseIndex]
|
|
|
|
p.responseIndex++
|
|
|
|
return resp, nil
|
|
}
|
|
|
|
func (p *mockProxier) ResponseIndex() int {
|
|
return p.responseIndex
|
|
}
|