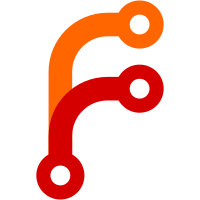
* vault-agent-cache: squashed 250+ commits * Add proper token revocation validations to the tests * Add more test cases * Avoid leaking by not closing request/response bodies; add comments * Fix revoke orphan use case; update tests * Add CLI test for making request over unix socket * agent/cache: remove namespace-related tests * Strip-off the auto-auth token from the lookup response * Output listener details along with configuration * Add scheme to API address output * leasecache: use IndexNameLease for prefix lease revocations * Make CLI accept the fully qualified unix address * export VAULT_AGENT_ADDR=unix://path/to/socket * unix:/ to unix://
29 lines
683 B
Go
29 lines
683 B
Go
package cache
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
|
|
"github.com/hashicorp/vault/api"
|
|
)
|
|
|
|
// SendRequest is the input for Proxier.Send.
|
|
type SendRequest struct {
|
|
Token string
|
|
Request *http.Request
|
|
RequestBody []byte
|
|
}
|
|
|
|
// SendResponse is the output from Proxier.Send.
|
|
type SendResponse struct {
|
|
Response *api.Response
|
|
ResponseBody []byte
|
|
}
|
|
|
|
// Proxier is the interface implemented by different components that are
|
|
// responsible for performing specific tasks, such as caching and proxying. All
|
|
// these tasks combined together would serve the request received by the agent.
|
|
type Proxier interface {
|
|
Send(ctx context.Context, req *SendRequest) (*SendResponse, error)
|
|
}
|