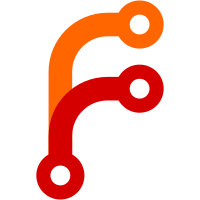
* adds ember-flight-icons dependecy * adds inline-json-import babel plugin * adds flight icon styling * updates Icon component to support flight icons * updates Icon component usages to new api and updates name values to flight icon set when available * fixes tests * updates icon story with flight mappings and fixes issue with flight icons not rendering in storybook * adds changelog * fixes typo in sign action glyph name in transit-key model * adds comments to icon-map * updates Icon component to use only supported flight icon sizes * adds icon transform codemod * updates icon transform formatting to handle edge case * runs icon transform on templates * updates Icon usage in toolbar-filter md and story * updates tests
38 lines
1.1 KiB
JavaScript
38 lines
1.1 KiB
JavaScript
/**
|
|
* @module ToolbarLink
|
|
* `ToolbarLink` components style links and buttons for the Toolbar
|
|
* It should only be used inside of `Toolbar`.
|
|
*
|
|
* @example
|
|
* ```js
|
|
* <Toolbar>
|
|
* <ToolbarActions>
|
|
* <ToolbarLink @params={{array 'vault.cluster.policies.create'}} @type="add" @disabled={{true}} @disabledTooltip="This link is disabled">
|
|
* Create policy
|
|
* </ToolbarLink>
|
|
* </ToolbarActions>
|
|
* </Toolbar>
|
|
* ```
|
|
*
|
|
* @param {array} params - Array to pass to LinkTo
|
|
* @param {string} type - Use "add" to change icon
|
|
* @param {boolean} disabled - pass true to disable link
|
|
* @param {string} disabledTooltip - tooltip to display on hover when disabled
|
|
*/
|
|
|
|
import Component from '@ember/component';
|
|
import { computed } from '@ember/object';
|
|
import layout from '../templates/components/toolbar-link';
|
|
|
|
export default Component.extend({
|
|
layout,
|
|
tagName: '',
|
|
supportsDataTestProperties: true,
|
|
type: null,
|
|
disabled: false,
|
|
disabledTooltip: null,
|
|
glyph: computed('type', function() {
|
|
return this.type == 'add' ? 'plus' : 'chevron-right';
|
|
}),
|
|
});
|