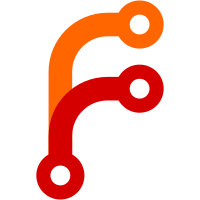
* runs ember-cli-update to 4.4.0 * updates yarn.lock * updates dependencies causing runtime errors (#17135) * Inject Store Service When Accessed Implicitly (#17345) * adds codemod for injecting store service * adds custom babylon parser with decorators-legacy plugin for jscodeshift transforms * updates inject-store-service codemod to only look for .extend object expressions and adds recast options * runs inject-store-service codemod on js files * replace query-params helper with hash (#17404) * Updates/removes dependencies throwing errors in Ember 4.4 (#17396) * updates ember-responsive to latest * updates ember-composable-helpers to latest and uses includes helper since contains was removed * updates ember-concurrency to latest * updates ember-cli-clipboard to latest * temporary workaround for toolbar-link component throwing errors for using params arg with LinkTo * adds missing store injection to auth configure route * fixes issue with string-list component throwing error for accessing prop in same computation * fixes non-iterable query params issue in mfa methods controller * refactors field-to-attrs to handle belongsTo rather than fragments * converts mount-config fragment to belongsTo on auth-method model * removes ember-api-actions and adds tune method to auth-method adapter * converts cluster replication attributes from fragment to relationship * updates ember-data, removes ember-data-fragments and updates yarn to latest * removes fragments from secret-engine model * removes fragment from test-form-model * removes commented out code * minor change to inject-store-service codemod and runs again on js files * Remove LinkTo positional params (#17421) * updates ember-cli-page-object to latest version * update toolbar-link to support link-to args and not positional params * adds replace arg to toolbar-link component * Clean up js lint errors (#17426) * replaces assert.equal to assert.strictEqual * update eslint no-console to error and disables invididual intended uses of console * cleans up hbs lint warnings (#17432) * Upgrade bug and test fixes (#17500) * updates inject-service codemod to take arg for service name and runs for flashMessages service * fixes hbs lint error after merging main * fixes flash messages * updates more deps * bug fixes * test fixes * updates ember-cli-content-security-policy and prevents default form submission throwing errors * more bug and test fixes * removes commented out code * fixes issue with code-mirror modifier sending change event on setup causing same computation error * Upgrade Clean Up (#17543) * updates deprecation workflow and filter * cleans up build errors, removes unused ivy-codemirror and sass and updates ember-cli-sass and node-sass to latest * fixes control groups test that was skipped after upgrade * updates control group service tests * addresses review feedback * updates control group service handleError method to use router.currentURL rather that transition.intent.url * adds changelog entry
99 lines
2.8 KiB
JavaScript
99 lines
2.8 KiB
JavaScript
/* eslint-env node */
|
|
'use strict';
|
|
|
|
const EmberApp = require('ember-cli/lib/broccoli/ember-app');
|
|
const config = require('./config/environment')();
|
|
const nodeSass = require('node-sass');
|
|
|
|
const environment = EmberApp.env();
|
|
const isProd = environment === 'production';
|
|
const isTest = environment === 'test';
|
|
// const isCI = !!process.env.CI;
|
|
|
|
const appConfig = {
|
|
'ember-service-worker': {
|
|
serviceWorkerScope: config.serviceWorkerScope,
|
|
skipWaitingOnMessage: true,
|
|
},
|
|
svgJar: {
|
|
//optimize: false,
|
|
//paths: [],
|
|
optimizer: {},
|
|
sourceDirs: ['node_modules/@hashicorp/structure-icons/dist', 'public'],
|
|
rootURL: '/ui/',
|
|
},
|
|
fingerprint: {
|
|
exclude: ['images/'],
|
|
},
|
|
assetLoader: {
|
|
generateURI: function (filePath) {
|
|
return `${config.rootURL.replace(/\/$/, '')}${filePath}`;
|
|
},
|
|
},
|
|
babel: {
|
|
plugins: [['inline-json-import', {}]],
|
|
},
|
|
hinting: isTest,
|
|
tests: isTest,
|
|
sourcemaps: {
|
|
enabled: !isProd,
|
|
},
|
|
sassOptions: {
|
|
implementation: nodeSass,
|
|
sourceMap: false,
|
|
onlyIncluded: true,
|
|
},
|
|
autoprefixer: {
|
|
enabled: isTest || isProd,
|
|
grid: true,
|
|
browsers: ['defaults'],
|
|
},
|
|
autoImport: {
|
|
forbidEval: true,
|
|
},
|
|
'ember-test-selectors': {
|
|
strip: isProd,
|
|
},
|
|
'ember-composable-helpers': {
|
|
except: ['array'],
|
|
},
|
|
'ember-cli-deprecation-workflow': {
|
|
enabled: true,
|
|
},
|
|
};
|
|
|
|
module.exports = function (defaults) {
|
|
let app = new EmberApp(defaults, appConfig);
|
|
|
|
app.import('vendor/string-includes.js');
|
|
app.import('node_modules/string.prototype.endswith/endswith.js');
|
|
app.import('node_modules/string.prototype.startswith/startswith.js');
|
|
|
|
app.import('node_modules/jsonlint/lib/jsonlint.js');
|
|
app.import('node_modules/codemirror/addon/lint/lint.css');
|
|
app.import('node_modules/codemirror/lib/codemirror.css');
|
|
app.import('node_modules/text-encoder-lite/text-encoder-lite.js');
|
|
app.import('node_modules/jsondiffpatch/dist/jsondiffpatch.umd.js');
|
|
app.import('node_modules/jsondiffpatch/dist/formatters-styles/html.css');
|
|
|
|
app.import('app/styles/bulma/bulma-radio-checkbox.css');
|
|
|
|
app.import('node_modules/@hashicorp/structure-icons/dist/loading.css');
|
|
app.import('node_modules/@hashicorp/structure-icons/dist/run.css');
|
|
|
|
// Use `app.import` to add additional libraries to the generated
|
|
// output files.
|
|
//
|
|
// If you need to use different assets in different
|
|
// environments, specify an object as the first parameter. That
|
|
// object's keys should be the environment name and the values
|
|
// should be the asset to use in that environment.
|
|
//
|
|
// If the library that you are including contains AMD or ES6
|
|
// modules that you would like to import into your application
|
|
// please specify an object with the list of modules as keys
|
|
// along with the exports of each module as its value.
|
|
|
|
return app.toTree();
|
|
};
|