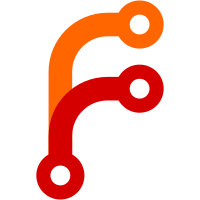
* Tackle #4929 a different way This turns c.sealed into an atomic, which allows us to call sealInternal without a lock. By doing so we can better control lock grabbing when a condition causing the standby loop to get out of active happens. This encapsulates that logic into two distinct pieces (although they could be combined into one), and makes lock guarding more understandable. * Re-add context canceling to the non-HA version of sealInternal * Return explicitly after stopCh triggered
126 lines
2.7 KiB
Go
126 lines
2.7 KiB
Go
package http
|
|
|
|
import (
|
|
"encoding/hex"
|
|
"net/http"
|
|
"reflect"
|
|
"testing"
|
|
|
|
"github.com/hashicorp/vault/vault"
|
|
)
|
|
|
|
func TestSysInit_get(t *testing.T) {
|
|
core := vault.TestCore(t)
|
|
ln, addr := TestServer(t, core)
|
|
defer ln.Close()
|
|
|
|
{
|
|
// Pre-init
|
|
resp, err := http.Get(addr + "/v1/sys/init")
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
var actual map[string]interface{}
|
|
expected := map[string]interface{}{
|
|
"initialized": false,
|
|
}
|
|
testResponseStatus(t, resp, 200)
|
|
testResponseBody(t, resp, &actual)
|
|
if !reflect.DeepEqual(actual, expected) {
|
|
t.Fatalf("bad: %#v", actual)
|
|
}
|
|
}
|
|
|
|
vault.TestCoreInit(t, core)
|
|
|
|
{
|
|
// Post-init
|
|
resp, err := http.Get(addr + "/v1/sys/init")
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
var actual map[string]interface{}
|
|
expected := map[string]interface{}{
|
|
"initialized": true,
|
|
}
|
|
testResponseStatus(t, resp, 200)
|
|
testResponseBody(t, resp, &actual)
|
|
if !reflect.DeepEqual(actual, expected) {
|
|
t.Fatalf("bad: %#v", actual)
|
|
}
|
|
}
|
|
}
|
|
|
|
// Test to check if the API errors out when wrong number of PGP keys are
|
|
// supplied
|
|
func TestSysInit_pgpKeysEntries(t *testing.T) {
|
|
core := vault.TestCore(t)
|
|
ln, addr := TestServer(t, core)
|
|
defer ln.Close()
|
|
|
|
resp := testHttpPut(t, "", addr+"/v1/sys/init", map[string]interface{}{
|
|
"secret_shares": 5,
|
|
"secret_threshold": 3,
|
|
"pgp_keys": []string{"pgpkey1"},
|
|
})
|
|
testResponseStatus(t, resp, 400)
|
|
}
|
|
|
|
// Test to check if the API errors out when wrong number of PGP keys are
|
|
// supplied for recovery config
|
|
func TestSysInit_pgpKeysEntriesForRecovery(t *testing.T) {
|
|
core := vault.TestCoreNewSeal(t)
|
|
ln, addr := TestServer(t, core)
|
|
defer ln.Close()
|
|
|
|
resp := testHttpPut(t, "", addr+"/v1/sys/init", map[string]interface{}{
|
|
"secret_shares": 1,
|
|
"secret_threshold": 1,
|
|
"stored_shares": 1,
|
|
"recovery_shares": 5,
|
|
"recovery_threshold": 3,
|
|
"recovery_pgp_keys": []string{"pgpkey1"},
|
|
})
|
|
testResponseStatus(t, resp, 400)
|
|
}
|
|
|
|
func TestSysInit_put(t *testing.T) {
|
|
core := vault.TestCore(t)
|
|
ln, addr := TestServer(t, core)
|
|
defer ln.Close()
|
|
|
|
resp := testHttpPut(t, "", addr+"/v1/sys/init", map[string]interface{}{
|
|
"secret_shares": 5,
|
|
"secret_threshold": 3,
|
|
})
|
|
|
|
var actual map[string]interface{}
|
|
testResponseStatus(t, resp, 200)
|
|
testResponseBody(t, resp, &actual)
|
|
keysRaw, ok := actual["keys"]
|
|
if !ok {
|
|
t.Fatalf("no keys: %#v", actual)
|
|
}
|
|
|
|
if _, ok := actual["root_token"]; !ok {
|
|
t.Fatal("no root token")
|
|
}
|
|
|
|
for _, key := range keysRaw.([]interface{}) {
|
|
keySlice, err := hex.DecodeString(key.(string))
|
|
if err != nil {
|
|
t.Fatalf("bad: %s", err)
|
|
}
|
|
|
|
if _, err := core.Unseal(keySlice); err != nil {
|
|
t.Fatalf("bad: %s", err)
|
|
}
|
|
}
|
|
|
|
if core.Sealed() {
|
|
t.Fatal("should not be sealed")
|
|
}
|
|
}
|