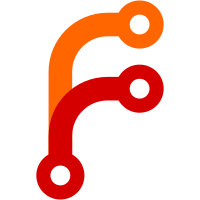
* work in progress: got the expired banner set with license check * wip: got the logic for both banners, need to test and write tests * add notes * prep for test writing * test coverage * add changelog * clean up * clarify dismissTypes and conditionals * updates * update comment * update comment * address pr comments * update test * small naming change * small naming changes * clean localStorage * comment clean up * another comment clean up * remove meep * add test coverage for new method in localStorage
66 lines
2.1 KiB
JavaScript
66 lines
2.1 KiB
JavaScript
import Component from '@glimmer/component';
|
|
import { action } from '@ember/object';
|
|
import { tracked } from '@glimmer/tracking';
|
|
import { inject as service } from '@ember/service';
|
|
import isAfter from 'date-fns/isAfter';
|
|
import differenceInDays from 'date-fns/differenceInDays';
|
|
import localStorage from 'vault/lib/local-storage';
|
|
|
|
/**
|
|
* @module LicenseBanners
|
|
* LicenseBanners components are used to display Vault-specific license expiry messages
|
|
*
|
|
* @example
|
|
* ```js
|
|
* <LicenseBanners @expiry={expiryDate} />
|
|
* ```
|
|
* @param {string} expiry - RFC3339 date timestamp
|
|
*/
|
|
|
|
export default class LicenseBanners extends Component {
|
|
@service version;
|
|
|
|
@tracked warningDismissed;
|
|
@tracked expiredDismissed;
|
|
|
|
constructor() {
|
|
super(...arguments);
|
|
// do not dismiss any banners if the user has updated their version
|
|
const dismissedBanner = localStorage.getItem(`dismiss-license-banner-${this.currentVersion}`); // returns either warning or expired
|
|
this.updateDismissType(dismissedBanner);
|
|
}
|
|
|
|
get currentVersion() {
|
|
return this.version.version;
|
|
}
|
|
|
|
get licenseExpired() {
|
|
if (!this.args.expiry) return false;
|
|
return isAfter(new Date(), new Date(this.args.expiry));
|
|
}
|
|
|
|
get licenseExpiringInDays() {
|
|
// Anything more than 30 does not render a warning
|
|
if (!this.args.expiry) return 99;
|
|
return differenceInDays(new Date(this.args.expiry), new Date());
|
|
}
|
|
|
|
@action
|
|
dismissBanner(dismissAction) {
|
|
// if a client's version changed their old localStorage key will still exists.
|
|
localStorage.cleanUpStorage('dismiss-license-banner', `dismiss-license-banner-${this.currentVersion}`);
|
|
// updates localStorage and then updates the template by calling updateDismissType
|
|
localStorage.setItem(`dismiss-license-banner-${this.currentVersion}`, dismissAction);
|
|
this.updateDismissType(dismissAction);
|
|
}
|
|
|
|
updateDismissType(dismissType) {
|
|
// updates tracked properties to update template
|
|
if (dismissType === 'warning') {
|
|
this.warningDismissed = true;
|
|
} else if (dismissType === 'expired') {
|
|
this.expiredDismissed = true;
|
|
}
|
|
}
|
|
}
|