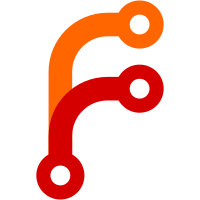
* add popups * add ability to disable entity and banner when entity is disabled * re-add alias-popup template * add accpetance tests for creating entities * add more entity creation acceptance tests * add delete to edit-form * add more identity tests and associated selectors * add onSuccess hook and use UnloadModel route mixins * add ability to toggle entity disabling from the popover * fix store list cache because unloadAll isn't synchronous * fill out tests for identity items and aliases * add ability to enable entity from the detail page * toArray on the peekAll * fix other tests/behavior that relied on a RecordArray * adjust layout for disabled entity and label for disabling an entity on the edit form * add item-details integration tests * move disable field on the entity form * use ghost buttons for delete in identity and policy edit forms * adding computed macros for lazy capability fetching and using them in the identity models
61 lines
1.6 KiB
JavaScript
61 lines
1.6 KiB
JavaScript
import Ember from 'ember';
|
|
import DS from 'ember-data';
|
|
import { TABS } from 'vault/helpers/tabs-for-identity-show';
|
|
|
|
export default Ember.Route.extend({
|
|
model(params) {
|
|
let { section } = params;
|
|
let itemType = this.modelFor('vault.cluster.access.identity');
|
|
let tabs = TABS[itemType];
|
|
let modelType = `identity/${itemType}`;
|
|
if (!tabs.includes(section)) {
|
|
const error = new DS.AdapterError();
|
|
Ember.set(error, 'httpStatus', 404);
|
|
throw error;
|
|
}
|
|
|
|
// if the record is in the store use that
|
|
let model = this.store.peekRecord(modelType, params.item_id);
|
|
|
|
// if we don't have creationTime, we only have a partial model so reload
|
|
if (model && !model.get('creationTime')) {
|
|
model = model.reload();
|
|
}
|
|
|
|
// if there's no model, we need to fetch it
|
|
if (!model) {
|
|
model = this.store.findRecord(modelType, params.item_id);
|
|
}
|
|
|
|
return Ember.RSVP.hash({
|
|
model,
|
|
section,
|
|
});
|
|
},
|
|
|
|
activate() {
|
|
// if we're just entering the route, and it's not a hard reload
|
|
// reload to make sure we have the newest info
|
|
if (this.currentModel) {
|
|
Ember.run.next(() => {
|
|
this.controller.get('model').reload();
|
|
});
|
|
}
|
|
},
|
|
|
|
afterModel(resolvedModel) {
|
|
let { section, model } = resolvedModel;
|
|
if (model.get('identityType') === 'group' && model.get('type') === 'internal' && section === 'aliases') {
|
|
return this.transitionTo('vault.cluster.access.identity.show', model.id, 'details');
|
|
}
|
|
},
|
|
|
|
setupController(controller, resolvedModel) {
|
|
let { model, section } = resolvedModel;
|
|
controller.setProperties({
|
|
model,
|
|
section,
|
|
});
|
|
},
|
|
});
|