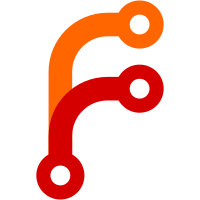
* Add priority queue to sdk * fix issue of storing pointers and now copy * update to use copy structure * Remove file, put Item struct def. into other file * add link * clean up docs * refactor internal data structure to hide heap method implementations. Other cleanup after feedback * rename PushItem and PopItem to just Push/Pop, after encapsulating the heap methods * updates after feedback * refactoring/renaming * guard against pushing a nil item * minor updates after feedback * Add SetCredentials, GenerateCredentials gRPC methods to combined database backend gPRC * Initial Combined database backend implementation of static accounts and automatic rotation * vendor updates * initial implementation of static accounts with Combined database backend, starting with PostgreSQL implementation * add lock and setup of rotation queue * vendor the queue * rebase on new method signature of queue * remove mongo tests for now * update default role sql * gofmt after rebase * cleanup after rebasing to remove checks for ErrNotFound error * rebase cdcr-priority-queue * vendor dependencies with 'go mod vendor' * website database docs for Static Role support * document the rotate-role API endpoint * postgres specific static role docs * use constants for paths * updates from review * remove dead code * combine and clarify error message for older plugins * Update builtin/logical/database/backend.go Co-Authored-By: Jim Kalafut <jim@kalafut.net> * cleanups from feedback * code and comment cleanups * move db.RLock higher to protect db.GenerateCredentials call * Return output with WALID if we failed to delete the WAL * Update builtin/logical/database/path_creds_create.go Co-Authored-By: Jim Kalafut <jim@kalafut.net> * updates after running 'make fmt' * update after running 'make proto' * Update builtin/logical/database/path_roles.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * Update builtin/logical/database/path_roles.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * update comment and remove and rearrange some dead code * Update website/source/api/secret/databases/index.html.md Co-Authored-By: Jim Kalafut <jim@kalafut.net> * cleanups after review * Update sdk/database/dbplugin/grpc_transport.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * code cleanup after feedback * remove PasswordLastSet; it's not used * document GenerateCredentials and SetCredentials * Update builtin/logical/database/path_rotate_credentials.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * wrap pop and popbykey in backend methods to protect against nil cred rotation queue * use strings.HasPrefix instead of direct equality check for path * Forgot to commit this * updates after feedback * re-purpose an outdated test to now check that static and dynamic roles cannot share a name * check for unique name across dynamic and static roles * refactor loadStaticWALs to return a map of name/setCredentialsWAL struct to consolidate where we're calling set credentials * remove commented out code * refactor to have loadstaticwals filter out wals for roles that no longer exist * return error if nil input given * add nil check for input into setStaticAccount * Update builtin/logical/database/path_roles.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * add constant for queue tick time in seconds, used for comparrison in updates * Update builtin/logical/database/path_roles.go Co-Authored-By: Jim Kalafut <jim@kalafut.net> * code cleanup after review * remove misplaced code comment * remove commented out code * create a queue in the Factory method, even if it's never used * update path_roles to use a common set of fields, with specific overrides for dynamic/static roles by type * document new method * move rotation things into a specific file * rename test file and consolidate some static account tests * Update builtin/logical/database/path_roles.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * Update builtin/logical/database/rotation.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * Update builtin/logical/database/rotation.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * Update builtin/logical/database/rotation.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * Update builtin/logical/database/rotation.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * Update builtin/logical/database/rotation.go Co-Authored-By: Brian Kassouf <briankassouf@users.noreply.github.com> * update code comments, method names, and move more methods into rotation.go * update comments to be capitalized * remove the item from the queue before we try to destroy it * findStaticWAL returns an error * use lowercase keys when encoding WAL entries * small cleanups * remove vestigial static account check * remove redundant DeleteWAL call in populate queue * if we error on loading role, push back to queue with 10 second backoff * poll in initqueue to make sure the backend is setup and can write/delete data * add revoke_user_on_delete flag to allow users to opt-in to revoking the static database user on delete of the Vault role. Default false * add code comments on read-only loop * code comment updates * re-push if error returned from find static wal * add locksutil and acquire locks when pop'ing from the queue * grab exclusive locks for updating static roles * Add SetCredentials and GenerateCredentials stubs to mockPlugin * add a switch in initQueue to listen for cancelation * remove guard on zero time, it should have no affect * create a new context in Factory to pass on and use for closing the backend queue * restore master copy of vendor dir
270 lines
6.9 KiB
Go
270 lines
6.9 KiB
Go
package dbplugin_test
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"os"
|
|
"testing"
|
|
"time"
|
|
|
|
log "github.com/hashicorp/go-hclog"
|
|
"github.com/hashicorp/vault/api"
|
|
"github.com/hashicorp/vault/helper/namespace"
|
|
vaulthttp "github.com/hashicorp/vault/http"
|
|
"github.com/hashicorp/vault/sdk/database/dbplugin"
|
|
"github.com/hashicorp/vault/sdk/helper/consts"
|
|
"github.com/hashicorp/vault/sdk/helper/pluginutil"
|
|
"github.com/hashicorp/vault/sdk/logical"
|
|
"github.com/hashicorp/vault/vault"
|
|
)
|
|
|
|
type mockPlugin struct {
|
|
users map[string][]string
|
|
}
|
|
|
|
var _ dbplugin.Database = &mockPlugin{}
|
|
|
|
func (m *mockPlugin) Type() (string, error) { return "mock", nil }
|
|
func (m *mockPlugin) CreateUser(_ context.Context, statements dbplugin.Statements, usernameConf dbplugin.UsernameConfig, expiration time.Time) (username string, password string, err error) {
|
|
err = errors.New("err")
|
|
if usernameConf.DisplayName == "" || expiration.IsZero() {
|
|
return "", "", err
|
|
}
|
|
|
|
if _, ok := m.users[usernameConf.DisplayName]; ok {
|
|
return "", "", err
|
|
}
|
|
|
|
m.users[usernameConf.DisplayName] = []string{password}
|
|
|
|
return usernameConf.DisplayName, "test", nil
|
|
}
|
|
func (m *mockPlugin) RenewUser(_ context.Context, statements dbplugin.Statements, username string, expiration time.Time) error {
|
|
err := errors.New("err")
|
|
if username == "" || expiration.IsZero() {
|
|
return err
|
|
}
|
|
|
|
if _, ok := m.users[username]; !ok {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|
|
func (m *mockPlugin) RevokeUser(_ context.Context, statements dbplugin.Statements, username string) error {
|
|
err := errors.New("err")
|
|
if username == "" {
|
|
return err
|
|
}
|
|
|
|
if _, ok := m.users[username]; !ok {
|
|
return err
|
|
}
|
|
|
|
delete(m.users, username)
|
|
return nil
|
|
}
|
|
func (m *mockPlugin) RotateRootCredentials(_ context.Context, statements []string) (map[string]interface{}, error) {
|
|
return nil, nil
|
|
}
|
|
func (m *mockPlugin) Init(_ context.Context, conf map[string]interface{}, _ bool) (map[string]interface{}, error) {
|
|
err := errors.New("err")
|
|
if len(conf) != 1 {
|
|
return nil, err
|
|
}
|
|
|
|
return conf, nil
|
|
}
|
|
func (m *mockPlugin) Initialize(_ context.Context, conf map[string]interface{}, _ bool) error {
|
|
err := errors.New("err")
|
|
if len(conf) != 1 {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|
|
func (m *mockPlugin) Close() error {
|
|
m.users = nil
|
|
return nil
|
|
}
|
|
|
|
func (m *mockPlugin) GenerateCredentials(ctx context.Context) (password string, err error) {
|
|
return password, err
|
|
}
|
|
|
|
func (m *mockPlugin) SetCredentials(ctx context.Context, statements dbplugin.Statements, staticConfig dbplugin.StaticUserConfig) (username string, password string, err error) {
|
|
return username, password, err
|
|
}
|
|
|
|
func getCluster(t *testing.T) (*vault.TestCluster, logical.SystemView) {
|
|
cluster := vault.NewTestCluster(t, nil, &vault.TestClusterOptions{
|
|
HandlerFunc: vaulthttp.Handler,
|
|
})
|
|
cluster.Start()
|
|
cores := cluster.Cores
|
|
|
|
sys := vault.TestDynamicSystemView(cores[0].Core)
|
|
vault.TestAddTestPlugin(t, cores[0].Core, "test-plugin", consts.PluginTypeDatabase, "TestPlugin_GRPC_Main", []string{}, "")
|
|
|
|
return cluster, sys
|
|
}
|
|
|
|
// This is not an actual test case, it's a helper function that will be executed
|
|
// by the go-plugin client via an exec call.
|
|
func TestPlugin_GRPC_Main(t *testing.T) {
|
|
if os.Getenv(pluginutil.PluginUnwrapTokenEnv) == "" {
|
|
return
|
|
}
|
|
|
|
plugin := &mockPlugin{
|
|
users: make(map[string][]string),
|
|
}
|
|
|
|
args := []string{"--tls-skip-verify=true"}
|
|
|
|
apiClientMeta := &api.PluginAPIClientMeta{}
|
|
flags := apiClientMeta.FlagSet()
|
|
flags.Parse(args)
|
|
|
|
dbplugin.Serve(plugin, api.VaultPluginTLSProvider(apiClientMeta.GetTLSConfig()))
|
|
}
|
|
|
|
func TestPlugin_Init(t *testing.T) {
|
|
cluster, sys := getCluster(t)
|
|
defer cluster.Cleanup()
|
|
|
|
dbRaw, err := dbplugin.PluginFactory(namespace.RootContext(nil), "test-plugin", sys, log.NewNullLogger())
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
connectionDetails := map[string]interface{}{
|
|
"test": 1,
|
|
}
|
|
|
|
_, err = dbRaw.Init(context.Background(), connectionDetails, true)
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
err = dbRaw.Close()
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
}
|
|
|
|
func TestPlugin_CreateUser(t *testing.T) {
|
|
cluster, sys := getCluster(t)
|
|
defer cluster.Cleanup()
|
|
|
|
db, err := dbplugin.PluginFactory(namespace.RootContext(nil), "test-plugin", sys, log.NewNullLogger())
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
defer db.Close()
|
|
|
|
connectionDetails := map[string]interface{}{
|
|
"test": 1,
|
|
}
|
|
|
|
_, err = db.Init(context.Background(), connectionDetails, true)
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
usernameConf := dbplugin.UsernameConfig{
|
|
DisplayName: "test",
|
|
RoleName: "test",
|
|
}
|
|
|
|
us, pw, err := db.CreateUser(context.Background(), dbplugin.Statements{}, usernameConf, time.Now().Add(time.Minute))
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
if us != "test" || pw != "test" {
|
|
t.Fatal("expected username and password to be 'test'")
|
|
}
|
|
|
|
// try and save the same user again to verify it saved the first time, this
|
|
// should return an error
|
|
_, _, err = db.CreateUser(context.Background(), dbplugin.Statements{}, usernameConf, time.Now().Add(time.Minute))
|
|
if err == nil {
|
|
t.Fatal("expected an error, user wasn't created correctly")
|
|
}
|
|
}
|
|
|
|
func TestPlugin_RenewUser(t *testing.T) {
|
|
cluster, sys := getCluster(t)
|
|
defer cluster.Cleanup()
|
|
|
|
db, err := dbplugin.PluginFactory(namespace.RootContext(nil), "test-plugin", sys, log.NewNullLogger())
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
defer db.Close()
|
|
|
|
connectionDetails := map[string]interface{}{
|
|
"test": 1,
|
|
}
|
|
_, err = db.Init(context.Background(), connectionDetails, true)
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
usernameConf := dbplugin.UsernameConfig{
|
|
DisplayName: "test",
|
|
RoleName: "test",
|
|
}
|
|
|
|
us, _, err := db.CreateUser(context.Background(), dbplugin.Statements{}, usernameConf, time.Now().Add(time.Minute))
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
err = db.RenewUser(context.Background(), dbplugin.Statements{}, us, time.Now().Add(time.Minute))
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
}
|
|
|
|
func TestPlugin_RevokeUser(t *testing.T) {
|
|
cluster, sys := getCluster(t)
|
|
defer cluster.Cleanup()
|
|
|
|
db, err := dbplugin.PluginFactory(namespace.RootContext(nil), "test-plugin", sys, log.NewNullLogger())
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
defer db.Close()
|
|
|
|
connectionDetails := map[string]interface{}{
|
|
"test": 1,
|
|
}
|
|
_, err = db.Init(context.Background(), connectionDetails, true)
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
usernameConf := dbplugin.UsernameConfig{
|
|
DisplayName: "test",
|
|
RoleName: "test",
|
|
}
|
|
|
|
us, _, err := db.CreateUser(context.Background(), dbplugin.Statements{}, usernameConf, time.Now().Add(time.Minute))
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
// Test default revoke statements
|
|
err = db.RevokeUser(context.Background(), dbplugin.Statements{}, us)
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
|
|
// Try adding the same username back so we can verify it was removed
|
|
_, _, err = db.CreateUser(context.Background(), dbplugin.Statements{}, usernameConf, time.Now().Add(time.Minute))
|
|
if err != nil {
|
|
t.Fatalf("err: %s", err)
|
|
}
|
|
}
|