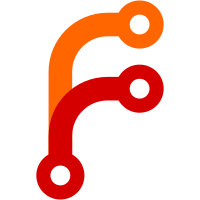
* add no-jquery rule and move event listeners to ember-concurrency tasks * remove unnecessary onchange and handleKeyDown actions * add element.closest polyfill and convert linked-block to use native dom apis * update pretender, fetch, page-object, add optional-features, remove ember/jquery * turn off jquery inclusion * remove jQuery.isPlainObject usage * violatedDirective isn't always formatted the same * use fetch and the ember-fetch adapter mixin * move to fetch and lowercase headers for pretender * display non-ember-data errors * use new async fn test style and lowercase headers in auth service test * setContext is not necessary with the new style tests and ember-cli-page-object - it actually triggers jquery usage * update ember-fetch, ember-cli-pretender * wait for permissions check * lowercase header name in auth test * refactor transit tests to one test per key type * simplify pollCluster helper * stop flakey tests by prefering the native fetch * avoid uncaught TransitionAborted error by navigating directly to unseal * unset model on controller after unloading it because controllers are singletons * update yarn.lock
26 lines
656 B
JavaScript
26 lines
656 B
JavaScript
import Mixin from '@ember/object/mixin';
|
|
|
|
// removes Ember Data records from the cache when the model
|
|
// changes or you move away from the current route
|
|
export default Mixin.create({
|
|
modelPath: 'model',
|
|
unloadModel() {
|
|
let { modelPath } = this;
|
|
let model = this.controller.get(modelPath);
|
|
if (!model || !model.unloadRecord) {
|
|
return;
|
|
}
|
|
this.store.unloadRecord(model);
|
|
model.destroy();
|
|
// it's important to unset the model on the controller since controllers are singletons
|
|
this.controller.set(modelPath, null);
|
|
},
|
|
|
|
actions: {
|
|
willTransition() {
|
|
this.unloadModel();
|
|
return true;
|
|
},
|
|
},
|
|
});
|