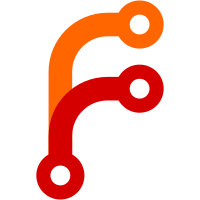
Add locking around UseToken and Lookup. Have UseToken flag an entry that needs to be revoked so that it can be done at the appropriate time, but so that Lookup in the interm doesn't return a value. The locking is a map of 4096 locks keyed off of the first three characters of the token ID which should provide good distribution.
42 lines
743 B
Go
42 lines
743 B
Go
package meta
|
|
|
|
import (
|
|
"flag"
|
|
"reflect"
|
|
"sort"
|
|
"testing"
|
|
)
|
|
|
|
func TestFlagSet(t *testing.T) {
|
|
cases := []struct {
|
|
Flags FlagSetFlags
|
|
Expected []string
|
|
}{
|
|
{
|
|
FlagSetNone,
|
|
[]string{},
|
|
},
|
|
{
|
|
FlagSetServer,
|
|
[]string{"address", "ca-cert", "ca-path", "client-cert", "client-key", "insecure", "tls-skip-verify", "wrap-ttl"},
|
|
},
|
|
}
|
|
|
|
for i, tc := range cases {
|
|
var m Meta
|
|
fs := m.FlagSet("foo", tc.Flags)
|
|
|
|
actual := make([]string, 0, 0)
|
|
fs.VisitAll(func(f *flag.Flag) {
|
|
actual = append(actual, f.Name)
|
|
})
|
|
sort.Strings(actual)
|
|
sort.Strings(tc.Expected)
|
|
|
|
if !reflect.DeepEqual(actual, tc.Expected) {
|
|
t.Fatalf("%d: flags: %#v\n\nExpected: %#v\nGot: %#v",
|
|
i, tc.Flags, tc.Expected, actual)
|
|
}
|
|
}
|
|
}
|