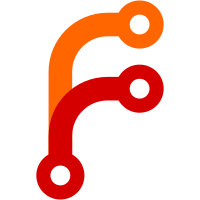
Our E2E "framework" has a bunch of features around test discovery and standing up infra that were never completed or fully used, and we ended up building out a large test suite that ignored all that in lieu of Terraform-provided infrastructure for the last couple years. This changeset is a proposal (and demonstration) for gradually migrating our E2E tests off the framework code so that developers can write fairly ordinary golang stdlib testing tests.
36 lines
1.1 KiB
Go
36 lines
1.1 KiB
Go
package e2eutil
|
|
|
|
import (
|
|
"testing"
|
|
|
|
capi "github.com/hashicorp/consul/api"
|
|
napi "github.com/hashicorp/nomad/api"
|
|
vapi "github.com/hashicorp/vault/api"
|
|
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
// NomadClient creates a default Nomad client based on the env vars
|
|
// from the test environment. Fails the test if it can't be created
|
|
func NomadClient(t *testing.T) *napi.Client {
|
|
client, err := napi.NewClient(napi.DefaultConfig())
|
|
require.NoError(t, err, "could not create Nomad client")
|
|
return client
|
|
}
|
|
|
|
// ConsulClient creates a default Consul client based on the env vars
|
|
// from the test environment. Fails the test if it can't be created
|
|
func ConsulClient(t *testing.T) *capi.Client {
|
|
client, err := capi.NewClient(capi.DefaultConfig())
|
|
require.NoError(t, err, "could not create Consul client")
|
|
return client
|
|
}
|
|
|
|
// VaultClient creates a default Vault client based on the env vars
|
|
// from the test environment. Fails the test if it can't be created
|
|
func VaultClient(t *testing.T) *vapi.Client {
|
|
client, err := vapi.NewClient(vapi.DefaultConfig())
|
|
require.NoError(t, err, "could not create Vault client")
|
|
return client
|
|
}
|