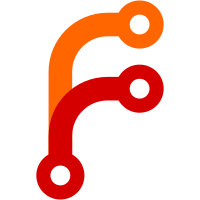
This updates the UI to use the new fuzzy search API. It’s a drop-in replacement so the / shortcut to jump to search is preserved, and results can be cycled through and chosen via arrow keys and the enter key. It doesn’t use everything returned by the API: * deployments and evaluations: these match by id, doesn’t seem like people would know those or benefit from quick navigation to them * namespaces: doesn’t seem useful as they currently function * scaling policies * tasks: the response doesn’t include an allocation id, which means they can’t be navigated to in the UI without an additional query * CSI volumes: aren’t actually returned by the API Since there’s no API to check the server configuration and know whether the feature has been disabled, this adds another query in route:application#beforeModel that acts as feature detection: if the attempt to query fails (500), the global search field is hidden. Upon having added another query on load, I realised that beforeModel was being triggered any time service:router#transitionTo was being called, which happens upon navigating to a search result, for instance, because of refreshModel being present on the region query parameter. This PR adds a check for transition.queryParamsOnly and skips rerunning the onload queries (token permissions check, license check, fuzzy search feature detection). Implementation notes: * there are changes to unrelated tests to ignore the on-load feature detection query * some lifecycle-related guards against undefined were required to address failures when navigating to an allocation * the minimum search length of 2 characters is hard-coded as there’s currently no way to determine min_term_length in the UI
105 lines
2.7 KiB
JavaScript
105 lines
2.7 KiB
JavaScript
/* eslint-disable ember/no-observers */
|
|
import Controller from '@ember/controller';
|
|
import { inject as service } from '@ember/service';
|
|
import { action, computed } from '@ember/object';
|
|
import { observes } from '@ember-decorators/object';
|
|
import { computed as overridable } from 'ember-overridable-computed';
|
|
import { alias } from '@ember/object/computed';
|
|
import { task } from 'ember-concurrency';
|
|
import Sortable from 'nomad-ui/mixins/sortable';
|
|
import { lazyClick } from 'nomad-ui/helpers/lazy-click';
|
|
import { watchRecord } from 'nomad-ui/utils/properties/watch';
|
|
import messageForError from 'nomad-ui/utils/message-from-adapter-error';
|
|
import classic from 'ember-classic-decorator';
|
|
|
|
@classic
|
|
export default class IndexController extends Controller.extend(Sortable) {
|
|
@service token;
|
|
|
|
queryParams = [
|
|
{
|
|
sortProperty: 'sort',
|
|
},
|
|
{
|
|
sortDescending: 'desc',
|
|
},
|
|
];
|
|
|
|
sortProperty = 'name';
|
|
sortDescending = false;
|
|
|
|
@alias('model.states') listToSort;
|
|
@alias('listSorted') sortedStates;
|
|
|
|
// Set in the route
|
|
preempter = null;
|
|
|
|
@overridable(function() {
|
|
// { title, description }
|
|
return null;
|
|
})
|
|
error;
|
|
|
|
@computed('model.allocatedResources.ports.@each.label')
|
|
get ports() {
|
|
return (this.get('model.allocatedResources.ports') || []).sortBy('label');
|
|
}
|
|
|
|
@computed('model.taskGroup.services.@each.name')
|
|
get services() {
|
|
return (this.get('model.taskGroup.services') || []).sortBy('name');
|
|
}
|
|
|
|
onDismiss() {
|
|
this.set('error', null);
|
|
}
|
|
|
|
@watchRecord('allocation') watchNext;
|
|
|
|
@observes('model.nextAllocation.clientStatus')
|
|
observeWatchNext() {
|
|
const nextAllocation = this.model.nextAllocation;
|
|
if (nextAllocation && nextAllocation.content) {
|
|
this.watchNext.perform(nextAllocation);
|
|
} else {
|
|
this.watchNext.cancelAll();
|
|
}
|
|
}
|
|
|
|
@task(function*() {
|
|
try {
|
|
yield this.model.stop();
|
|
// Eagerly update the allocation clientStatus to avoid flickering
|
|
this.model.set('clientStatus', 'complete');
|
|
} catch (err) {
|
|
this.set('error', {
|
|
title: 'Could Not Stop Allocation',
|
|
description: messageForError(err, 'manage allocation lifecycle'),
|
|
});
|
|
}
|
|
})
|
|
stopAllocation;
|
|
|
|
@task(function*() {
|
|
try {
|
|
yield this.model.restart();
|
|
} catch (err) {
|
|
this.set('error', {
|
|
title: 'Could Not Restart Allocation',
|
|
description: messageForError(err, 'manage allocation lifecycle'),
|
|
});
|
|
}
|
|
})
|
|
restartAllocation;
|
|
|
|
@action
|
|
gotoTask(allocation, task) {
|
|
this.transitionToRoute('allocations.allocation.task', task);
|
|
}
|
|
|
|
@action
|
|
taskClick(allocation, task, event) {
|
|
lazyClick([() => this.send('gotoTask', allocation, task), event]);
|
|
}
|
|
}
|