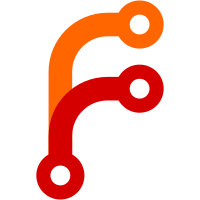
* Recursive trie-building with variable paths * tree structure applied to new path routes and a new util class * Breadcrumbs for SV paths and prompt when nothing exists at a path * Lint and test cleanup * Pre-review cleanup * lintfix * Abstracted pathtree each-ins into a new component class * Path tree component styles * Types added and PR feedback addressed * Path tree to variable paths * Slightly simpler path QP mods * More pr feedback handling * Trim moved into a function on variable model * Traversal and compaction tests for PathTree * Trim Path tests * Variable-paths component tests * Lint fixup for tests
48 lines
1 KiB
JavaScript
48 lines
1 KiB
JavaScript
import Component from '@glimmer/component';
|
|
import { action } from '@ember/object';
|
|
import { tracked } from '@glimmer/tracking';
|
|
import { inject as service } from '@ember/service';
|
|
export default class SecureVariableFormComponent extends Component {
|
|
@service router;
|
|
|
|
@tracked
|
|
shouldHideValues = true;
|
|
|
|
get valueFieldType() {
|
|
return this.shouldHideValues ? 'password' : 'text';
|
|
}
|
|
|
|
get shouldDisableSave() {
|
|
return !this.args.model?.path;
|
|
}
|
|
|
|
@action
|
|
toggleShowHide() {
|
|
this.shouldHideValues = !this.shouldHideValues;
|
|
}
|
|
|
|
@action appendRow() {
|
|
this.args.model.keyValues.pushObject({
|
|
key: '',
|
|
value: '',
|
|
});
|
|
}
|
|
|
|
@action deleteRow(row) {
|
|
this.args.model.keyValues.removeObject(row);
|
|
}
|
|
|
|
@action
|
|
async save(e) {
|
|
e.preventDefault();
|
|
this.args.model.setAndTrimPath();
|
|
|
|
const transitionTarget = this.args.model.isNew
|
|
? 'variables'
|
|
: 'variables.variable';
|
|
|
|
await this.args.model.save();
|
|
this.router.transitionTo(transitionTarget);
|
|
}
|
|
}
|