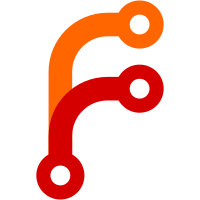
Now that tasks that have finished running can be restarted, the UI needs to use the actual task state to determine which CSS class to use when rendering the task lifecycle chart element.
44 lines
968 B
JavaScript
44 lines
968 B
JavaScript
import Component from '@ember/component';
|
|
import { computed } from '@ember/object';
|
|
import { tagName } from '@ember-decorators/component';
|
|
import classic from 'ember-classic-decorator';
|
|
|
|
@classic
|
|
@tagName('')
|
|
export default class LifecycleChartRow extends Component {
|
|
@computed('taskState.state')
|
|
get activeClass() {
|
|
if (this.taskState && this.taskState.state === 'running') {
|
|
return 'is-active';
|
|
}
|
|
|
|
return undefined;
|
|
}
|
|
|
|
@computed('taskState.state')
|
|
get finishedClass() {
|
|
if (this.taskState && this.taskState.state === 'dead') {
|
|
return 'is-finished';
|
|
}
|
|
|
|
return undefined;
|
|
}
|
|
|
|
@computed('task.lifecycleName')
|
|
get lifecycleLabel() {
|
|
if (!this.task) {
|
|
return '';
|
|
}
|
|
|
|
const name = this.task.lifecycleName;
|
|
|
|
if (name.includes('sidecar')) {
|
|
return 'sidecar';
|
|
} else if (name.includes('ephemeral')) {
|
|
return name.substr(0, name.indexOf('-'));
|
|
} else {
|
|
return name;
|
|
}
|
|
}
|
|
}
|