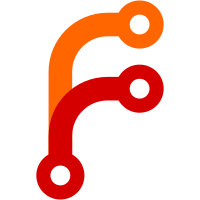
Also fixed the same typo in a test. Fixing the typo fixes the link, but the link was still broken when running the website locally due to the trailing slash. It would have worked in prod thanks to redirects, but using the canonical URL seems ideal.
66 lines
1.4 KiB
Go
66 lines
1.4 KiB
Go
package client
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/hashicorp/nomad/client/config"
|
|
"github.com/hashicorp/nomad/helper/uuid"
|
|
"github.com/hashicorp/nomad/nomad/structs"
|
|
"github.com/hashicorp/nomad/testutil"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestHeartbeatStop_allocHook(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
server, _, cleanupS1 := testServer(t, nil)
|
|
defer cleanupS1()
|
|
testutil.WaitForLeader(t, server.RPC)
|
|
|
|
client, cleanupC1 := TestClient(t, func(c *config.Config) {
|
|
c.RPCHandler = server
|
|
})
|
|
defer cleanupC1()
|
|
|
|
// an allocation, with a tiny lease
|
|
d := 1 * time.Microsecond
|
|
alloc := &structs.Allocation{
|
|
ID: uuid.Generate(),
|
|
TaskGroup: "foo",
|
|
Job: &structs.Job{
|
|
TaskGroups: []*structs.TaskGroup{
|
|
{
|
|
Name: "foo",
|
|
StopAfterClientDisconnect: &d,
|
|
},
|
|
},
|
|
},
|
|
Resources: &structs.Resources{
|
|
CPU: 100,
|
|
MemoryMB: 100,
|
|
DiskMB: 0,
|
|
},
|
|
}
|
|
|
|
// alloc added to heartbeatStop.allocs
|
|
err := client.addAlloc(alloc, "")
|
|
require.NoError(t, err)
|
|
testutil.WaitForResult(func() (bool, error) {
|
|
_, ok := client.heartbeatStop.allocInterval[alloc.ID]
|
|
return ok, nil
|
|
}, func(err error) {
|
|
require.NoError(t, err)
|
|
})
|
|
|
|
// the tiny lease causes the watch loop to destroy it
|
|
testutil.WaitForResult(func() (bool, error) {
|
|
_, ok := client.heartbeatStop.allocInterval[alloc.ID]
|
|
return !ok, nil
|
|
}, func(err error) {
|
|
require.NoError(t, err)
|
|
})
|
|
|
|
require.Empty(t, client.allocs[alloc.ID])
|
|
}
|