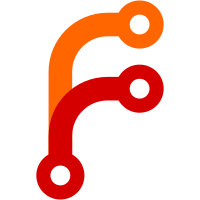
This PR switches the Nomad repository from using govendor to Go modules for managing dependencies. Aspects of the Nomad workflow remain pretty much the same. The usual Makefile targets should continue to work as they always did. The API submodule simply defers to the parent Nomad version on the repository, keeping the semantics of API versioning that currently exists.
78 lines
1.9 KiB
Go
78 lines
1.9 KiB
Go
package ecs
|
|
|
|
import (
|
|
"github.com/denverdino/aliyungo/common"
|
|
"github.com/denverdino/aliyungo/util"
|
|
)
|
|
|
|
type DescribeVRoutersArgs struct {
|
|
VRouterId string
|
|
RegionId common.Region
|
|
common.Pagination
|
|
}
|
|
|
|
//
|
|
// You can read doc at http://docs.aliyun.com/#/pub/ecs/open-api/datatype&vroutersettype
|
|
type VRouterSetType struct {
|
|
VRouterId string
|
|
RegionId common.Region
|
|
VpcId string
|
|
RouteTableIds struct {
|
|
RouteTableId []string
|
|
}
|
|
VRouterName string
|
|
Description string
|
|
CreationTime util.ISO6801Time
|
|
}
|
|
|
|
type DescribeVRoutersResponse struct {
|
|
common.Response
|
|
common.PaginationResult
|
|
VRouters struct {
|
|
VRouter []VRouterSetType
|
|
}
|
|
}
|
|
|
|
// DescribeVRouters describes Virtual Routers
|
|
//
|
|
// You can read doc at http://docs.aliyun.com/#/pub/ecs/open-api/vrouter&describevrouters
|
|
func (client *Client) DescribeVRouters(args *DescribeVRoutersArgs) (vrouters []VRouterSetType, pagination *common.PaginationResult, err error) {
|
|
response, err := client.DescribeVRoutersWithRaw(args)
|
|
if err == nil {
|
|
return response.VRouters.VRouter, &response.PaginationResult, nil
|
|
}
|
|
|
|
return nil, nil, err
|
|
}
|
|
|
|
func (client *Client) DescribeVRoutersWithRaw(args *DescribeVRoutersArgs) (response *DescribeVRoutersResponse, err error) {
|
|
args.Validate()
|
|
response = &DescribeVRoutersResponse{}
|
|
|
|
err = client.Invoke("DescribeVRouters", args, response)
|
|
|
|
if err == nil {
|
|
return response, nil
|
|
}
|
|
|
|
return nil, err
|
|
}
|
|
|
|
type ModifyVRouterAttributeArgs struct {
|
|
VRouterId string
|
|
VRouterName string
|
|
Description string
|
|
}
|
|
|
|
type ModifyVRouterAttributeResponse struct {
|
|
common.Response
|
|
}
|
|
|
|
// ModifyVRouterAttribute modifies attribute of Virtual Router
|
|
//
|
|
// You can read doc at http://docs.aliyun.com/#/pub/ecs/open-api/vrouter&modifyvrouterattribute
|
|
func (client *Client) ModifyVRouterAttribute(args *ModifyVRouterAttributeArgs) error {
|
|
response := ModifyVRouterAttributeResponse{}
|
|
return client.Invoke("ModifyVRouterAttribute", args, &response)
|
|
}
|