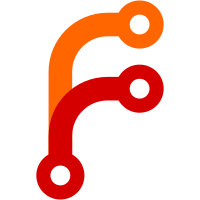
* Starting on namespaced id * Traversal for variables uniqued by namespace * Delog * Basic CRUD complete w namespaces included * Correct secvar breadcrumb joining and testfix now that namespaces are included * Testfixes with namespaces in place * Namespace-aware duplicate path warning * Duplicate path warning test additions * Trimpath reimplemented on dupe check * Solves a bug where slash was not being passed to the can write check * PR fixes * variable paths integration test fix now uses store * Seems far less hacky in retrospect * PR feedback addressed * test fixes after inclusion of path as local non-model var * Prevent confusion by dropping namespace from QPs on PUT, since its already in .data * Solves a harsh bug where you have namespace access but no secvars access (#14098) * Solves a harsh bug where you have namespace access but no secvars access * Lint cleanup * Remove unneeded condition
53 lines
1.1 KiB
JavaScript
53 lines
1.1 KiB
JavaScript
import Controller, { inject as controller } from '@ember/controller';
|
|
import { action } from '@ember/object';
|
|
|
|
const ALL_NAMESPACE_WILDCARD = '*';
|
|
|
|
export default class VariablesPathController extends Controller {
|
|
get absolutePath() {
|
|
return this.model?.absolutePath || '';
|
|
}
|
|
get breadcrumbs() {
|
|
if (this.absolutePath) {
|
|
let crumbs = [];
|
|
this.absolutePath.split('/').reduce((m, n) => {
|
|
crumbs.push({
|
|
label: n,
|
|
args: [`variables.path`, m + n],
|
|
});
|
|
return m + n + '/';
|
|
}, []);
|
|
return crumbs;
|
|
} else {
|
|
return [];
|
|
}
|
|
}
|
|
|
|
@controller variables;
|
|
|
|
@action
|
|
setNamespace(namespace) {
|
|
this.variables.setNamespace(namespace);
|
|
}
|
|
|
|
get namespaceSelection() {
|
|
return this.variables.qpNamespace;
|
|
}
|
|
|
|
get namespaceOptions() {
|
|
const namespaces = this.store
|
|
.peekAll('namespace')
|
|
.map(({ name }) => ({ key: name, label: name }));
|
|
|
|
if (namespaces.length <= 1) return null;
|
|
|
|
// Create default namespace selection
|
|
namespaces.unshift({
|
|
key: ALL_NAMESPACE_WILDCARD,
|
|
label: 'All (*)',
|
|
});
|
|
|
|
return namespaces;
|
|
}
|
|
}
|