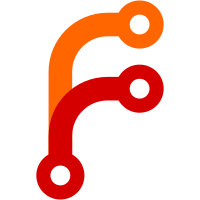
This change introduces the Task API: a portable way for tasks to access Nomad's HTTP API. This particular implementation uses a Unix Domain Socket and, unlike the agent's HTTP API, always requires authentication even if ACLs are disabled. This PR contains the core feature and tests but followup work is required for the following TODO items: - Docs - might do in a followup since dynamic node metadata / task api / workload id all need to interlink - Unit tests for auth middleware - Caching for auth middleware - Rate limiting on negative lookups for auth middleware --------- Co-authored-by: Seth Hoenig <shoenig@duck.com>
68 lines
1.8 KiB
Go
68 lines
1.8 KiB
Go
//go:build windows
|
|
|
|
package users
|
|
|
|
import (
|
|
"os"
|
|
"os/user"
|
|
"path/filepath"
|
|
"testing"
|
|
|
|
"github.com/shoenig/test/must"
|
|
)
|
|
|
|
func TestLookup_Windows(t *testing.T) {
|
|
stdlibUser, err := user.Current()
|
|
must.NoError(t, err, must.Sprintf("error looking up current user using stdlib"))
|
|
must.NotEq(t, "", stdlibUser.Username)
|
|
|
|
helperUser, err := Current()
|
|
must.NoError(t, err)
|
|
|
|
must.Eq(t, stdlibUser.Username, helperUser.Username)
|
|
|
|
lookupUser, err := Lookup(helperUser.Username)
|
|
must.NoError(t, err)
|
|
|
|
must.Eq(t, helperUser.Username, lookupUser.Username)
|
|
}
|
|
|
|
func TestLookup_Administrator(t *testing.T) {
|
|
u, err := user.Lookup("Administrator")
|
|
must.NoError(t, err)
|
|
|
|
// Windows allows looking up unqualified names but will return a fully
|
|
// qualified (eg prefixed with the local machine or domain)
|
|
must.StrHasSuffix(t, "Administrator", u.Username)
|
|
}
|
|
|
|
func TestWriteFileFor_Windows(t *testing.T) {
|
|
path := filepath.Join(t.TempDir(), "secret.txt")
|
|
contents := []byte("TOO MANY SECRETS")
|
|
|
|
must.NoError(t, WriteFileFor(path, contents, "Administrator"))
|
|
stat, err := os.Lstat(path)
|
|
must.NoError(t, err)
|
|
must.True(t, stat.Mode().IsRegular(),
|
|
must.Sprintf("expected %s to be a regular file but found %#o", path, stat.Mode()))
|
|
|
|
// Assert Windows hits the fallback world-accessible case
|
|
must.Eq(t, 0o666, stat.Mode().Perm())
|
|
}
|
|
|
|
// TestSocketFileFor_Windows asserts that socket files cannot be chowned on
|
|
// windows.
|
|
func TestSocketFileFor_Windows(t *testing.T) {
|
|
path := filepath.Join(t.TempDir(), "api.sock")
|
|
|
|
ln, err := SocketFileFor(testlog.HCLogger(t), path, "Administrator")
|
|
must.NoError(t, err)
|
|
must.NotNil(t, ln)
|
|
defer ln.Close()
|
|
stat, err := os.Lstat(path)
|
|
must.NoError(t, err)
|
|
|
|
// Assert Windows hits the fallback world-accessible case
|
|
must.Eq(t, 0o666, stat.Mode().Perm())
|
|
}
|