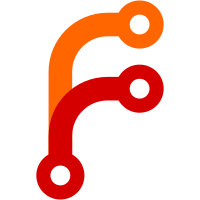
* Process to send events to configured sinks This PR adds a SinkManager to a server which is responsible for managing managed sinks. Managed sinks subscribe to the event broker and send events to a sink writer (webhook). When changes to the eventstore are made the sinkmanager and managed sink are responsible for reloading or starting a new managed sink. * periodically check in sink progress to raft Save progress on the last successfully sent index to raft. This allows a managed sink to resume close to where it left off in the event of a lost server or leadership change dereference eventsink so we can accurately use the watchch When using a pointer to eventsink struct it was updated immediately and our reload logic would not trigger
56 lines
1.1 KiB
Go
56 lines
1.1 KiB
Go
package stream
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
"net/http"
|
|
"net/http/httptest"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/hashicorp/nomad/nomad/mock"
|
|
"github.com/hashicorp/nomad/nomad/structs"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
var _ SinkWriter = &WebhookSink{}
|
|
|
|
func TestWebhookSink_Basic(t *testing.T) {
|
|
received := make(chan struct{})
|
|
|
|
ts := httptest.NewServer(http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) {
|
|
require.Equal(t, "application/json", r.Header.Get("Content-Type"))
|
|
|
|
var event structs.Events
|
|
dec := json.NewDecoder(r.Body)
|
|
require.NoError(t, dec.Decode(&event))
|
|
require.Equal(t, "Deployment", string(event.Events[0].Topic))
|
|
|
|
close(received)
|
|
}))
|
|
defer ts.Close()
|
|
|
|
sink := mock.EventSink()
|
|
sink.Address = ts.URL
|
|
|
|
webhook, err := NewWebhookSink(sink)
|
|
require.NoError(t, err)
|
|
|
|
e := &structs.Events{
|
|
Index: 1,
|
|
Events: []structs.Event{
|
|
{
|
|
Topic: "Deployment",
|
|
},
|
|
},
|
|
}
|
|
webhook.Send(context.Background(), e)
|
|
|
|
select {
|
|
case <-received:
|
|
// success
|
|
case <-time.After(2 * time.Second):
|
|
require.Fail(t, "expected test server to receive webhook")
|
|
}
|
|
}
|