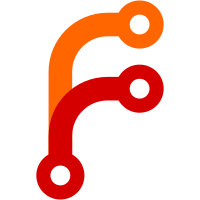
The e2e framework instantiates clients for Nomad/Consul but the provisioning of the actual Nomad cluster is left to Terraform. The Terraform provisioning process uses `remote-exec` to deploy specific versions of Nomad so that we don't have to bake an AMI every time we want to test a new version. But Terraform treats the resulting instances as immutable, so we can't use the same tooling to update the version of Nomad in-place. This is a prerequisite for upgrade testing. This changeset extends the e2e framework to provide the option of deploying Nomad (and, in the future, Consul/Vault) with specific versions to running infrastructure. This initial implementation is focused on deploying to a single cluster via `ssh` (because that's our current need), but provides interfaces to hook the test run at the start of the run, the start of each suite, or the start of a given test case. Terraform work includes: * provides Terraform output that written to JSON used by the framework to configure provisioning via `terraform output provisioning`. * provides Terraform output that can be used by test operators to configure their shell via `$(terraform output environment)` * drops `remote-exec` provisioning steps from Terraform * makes changes to the deployment scripts to ensure they can be run multiple times w/ different versions against the same host.
73 lines
2.2 KiB
Go
73 lines
2.2 KiB
Go
package provisioning
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"testing"
|
|
|
|
capi "github.com/hashicorp/consul/api"
|
|
napi "github.com/hashicorp/nomad/api"
|
|
"github.com/hashicorp/nomad/helper/uuid"
|
|
vapi "github.com/hashicorp/vault/api"
|
|
)
|
|
|
|
// DefaultProvisioner is a noop provisioner that builds clients from environment
|
|
// variables according to the respective client configuration
|
|
var DefaultProvisioner Provisioner = new(singleClusterProvisioner)
|
|
|
|
type singleClusterProvisioner struct{}
|
|
|
|
// SetupTestRun in the default case is a no-op.
|
|
func (p *singleClusterProvisioner) SetupTestRun(t *testing.T, opts SetupOptions) (*ClusterInfo, error) {
|
|
return &ClusterInfo{}, nil
|
|
}
|
|
|
|
// SetupTestSuite in the default case is a no-op.
|
|
func (p *singleClusterProvisioner) SetupTestSuite(t *testing.T, opts SetupOptions) (*ClusterInfo, error) {
|
|
return &ClusterInfo{}, nil
|
|
}
|
|
|
|
// SetupTestCase in the default case only creates new clients and embeds the
|
|
// TestCase name into the ClusterInfo handle.
|
|
func (p *singleClusterProvisioner) SetupTestCase(t *testing.T, opts SetupOptions) (*ClusterInfo, error) {
|
|
// Build ID based off given name
|
|
info := &ClusterInfo{
|
|
ID: uuid.Generate()[:8],
|
|
Name: opts.Name,
|
|
}
|
|
|
|
// Build Nomad api client
|
|
nomadClient, err := napi.NewClient(napi.DefaultConfig())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
info.NomadClient = nomadClient
|
|
|
|
if opts.ExpectConsul {
|
|
consulClient, err := capi.NewClient(capi.DefaultConfig())
|
|
if err != nil {
|
|
return nil, fmt.Errorf("expected Consul: %v", err)
|
|
}
|
|
info.ConsulClient = consulClient
|
|
}
|
|
|
|
if len(os.Getenv(vapi.EnvVaultAddress)) != 0 {
|
|
vaultClient, err := vapi.NewClient(vapi.DefaultConfig())
|
|
if err != nil && opts.ExpectVault {
|
|
return nil, err
|
|
}
|
|
info.VaultClient = vaultClient
|
|
} else if opts.ExpectVault {
|
|
return nil, fmt.Errorf("vault client expected but environment variable %s not set",
|
|
vapi.EnvVaultAddress)
|
|
}
|
|
|
|
return info, err
|
|
}
|
|
|
|
// all TearDown* methods of the default provisioner leave the test environment in place
|
|
|
|
func (p *singleClusterProvisioner) TearDownTestCase(_ *testing.T, _ string) error { return nil }
|
|
func (p *singleClusterProvisioner) TearDownTestSuite(_ *testing.T, _ string) error { return nil }
|
|
func (p *singleClusterProvisioner) TearDownTestRun(_ *testing.T, _ string) error { return nil }
|