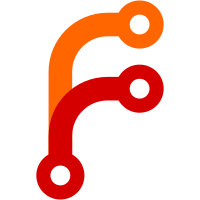
Nomad inherited protocol version numbering configuration from Consul and Serf, but unlike those projects Nomad has never used it. Nomad's `protocol_version` has always been `1`. While the code is effectively unused and therefore poses no runtime risks to leave, I felt like removing it was best because: 1. Nomad's RPC subsystem has been able to evolve extensively without needing to increment the version number. 2. Nomad's HTTP API has evolved extensively without increment `API{Major,Minor}Version`. If we want to version the HTTP API in the future, I doubt this is the mechanism we would choose. 3. The presence of the `server.protocol_version` configuration parameter is confusing since `server.raft_protocol` *is* an important parameter for operators to consider. Even more confusing is that there is a distinct Serf protocol version which is included in `nomad server members` output under the heading `Protocol`. `raft_protocol` is the *only* protocol version relevant to Nomad developers and operators. The other protocol versions are either deadcode or have never changed (Serf). 4. If we were to need to version the RPC, HTTP API, or Serf protocols, I don't think these configuration parameters and variables are the best choice. If we come to that point we should choose a versioning scheme based on the use case and modern best practices -- not this 6+ year old dead code.
133 lines
3.4 KiB
Go
133 lines
3.4 KiB
Go
package nomad
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"strconv"
|
|
|
|
log "github.com/hashicorp/go-hclog"
|
|
|
|
"github.com/hashicorp/consul/agent/consul/autopilot"
|
|
"github.com/hashicorp/nomad/nomad/structs"
|
|
)
|
|
|
|
// Status endpoint is used to check on server status
|
|
type Status struct {
|
|
srv *Server
|
|
logger log.Logger
|
|
}
|
|
|
|
// Ping is used to just check for connectivity
|
|
func (s *Status) Ping(args struct{}, reply *struct{}) error {
|
|
return nil
|
|
}
|
|
|
|
// Leader is used to get the address of the leader
|
|
func (s *Status) Leader(args *structs.GenericRequest, reply *string) error {
|
|
if args.Region == "" {
|
|
args.Region = s.srv.config.Region
|
|
}
|
|
if done, err := s.srv.forward("Status.Leader", args, args, reply); done {
|
|
return err
|
|
}
|
|
|
|
leader := string(s.srv.raft.Leader())
|
|
if leader != "" {
|
|
*reply = leader
|
|
} else {
|
|
*reply = ""
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Peers is used to get all the Raft peers
|
|
func (s *Status) Peers(args *structs.GenericRequest, reply *[]string) error {
|
|
if args.Region == "" {
|
|
args.Region = s.srv.config.Region
|
|
}
|
|
if done, err := s.srv.forward("Status.Peers", args, args, reply); done {
|
|
return err
|
|
}
|
|
|
|
future := s.srv.raft.GetConfiguration()
|
|
if err := future.Error(); err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, server := range future.Configuration().Servers {
|
|
*reply = append(*reply, string(server.Address))
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Members return the list of servers in a cluster that a particular server is
|
|
// aware of
|
|
func (s *Status) Members(args *structs.GenericRequest, reply *structs.ServerMembersResponse) error {
|
|
// Check node read permissions
|
|
if aclObj, err := s.srv.ResolveToken(args.AuthToken); err != nil {
|
|
return err
|
|
} else if aclObj != nil && !aclObj.AllowNodeRead() {
|
|
return structs.ErrPermissionDenied
|
|
}
|
|
|
|
serfMembers := s.srv.Members()
|
|
members := make([]*structs.ServerMember, len(serfMembers))
|
|
for i, mem := range serfMembers {
|
|
members[i] = &structs.ServerMember{
|
|
Name: mem.Name,
|
|
Addr: mem.Addr,
|
|
Port: mem.Port,
|
|
Tags: mem.Tags,
|
|
Status: mem.Status.String(),
|
|
ProtocolMin: mem.ProtocolMin,
|
|
ProtocolMax: mem.ProtocolMax,
|
|
ProtocolCur: mem.ProtocolCur,
|
|
DelegateMin: mem.DelegateMin,
|
|
DelegateMax: mem.DelegateMax,
|
|
DelegateCur: mem.DelegateCur,
|
|
}
|
|
}
|
|
*reply = structs.ServerMembersResponse{
|
|
ServerName: s.srv.config.NodeName,
|
|
ServerRegion: s.srv.config.Region,
|
|
ServerDC: s.srv.config.Datacenter,
|
|
Members: members,
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// RaftStats is used by Autopilot to query the raft stats of the local server.
|
|
func (s *Status) RaftStats(args struct{}, reply *autopilot.ServerStats) error {
|
|
stats := s.srv.raft.Stats()
|
|
|
|
var err error
|
|
reply.LastContact = stats["last_contact"]
|
|
reply.LastIndex, err = strconv.ParseUint(stats["last_log_index"], 10, 64)
|
|
if err != nil {
|
|
return fmt.Errorf("error parsing server's last_log_index value: %s", err)
|
|
}
|
|
reply.LastTerm, err = strconv.ParseUint(stats["last_log_term"], 10, 64)
|
|
if err != nil {
|
|
return fmt.Errorf("error parsing server's last_log_term value: %s", err)
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// HasNodeConn returns whether the server has a connection to the requested
|
|
// Node.
|
|
func (s *Status) HasNodeConn(args *structs.NodeSpecificRequest, reply *structs.NodeConnQueryResponse) error {
|
|
// Validate the args
|
|
if args.NodeID == "" {
|
|
return errors.New("Must provide the NodeID")
|
|
}
|
|
|
|
state, ok := s.srv.getNodeConn(args.NodeID)
|
|
if ok {
|
|
reply.Connected = true
|
|
reply.Established = state.Established
|
|
}
|
|
|
|
return nil
|
|
}
|