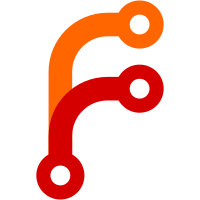
This followup to #10066 adds a step to clear the one-time token from the URL after the application has loaded. The delay is required for it to actually clear, but only when the OTT is present to avoid slowing down the entire test suite.
123 lines
3 KiB
JavaScript
123 lines
3 KiB
JavaScript
import { inject as service } from '@ember/service';
|
|
import { later, next } from '@ember/runloop';
|
|
import Route from '@ember/routing/route';
|
|
import { AbortError } from '@ember-data/adapter/error';
|
|
import RSVP from 'rsvp';
|
|
import { action } from '@ember/object';
|
|
import classic from 'ember-classic-decorator';
|
|
|
|
@classic
|
|
export default class ApplicationRoute extends Route {
|
|
@service config;
|
|
@service system;
|
|
@service store;
|
|
@service token;
|
|
|
|
queryParams = {
|
|
region: {
|
|
refreshModel: true,
|
|
},
|
|
};
|
|
|
|
resetController(controller, isExiting) {
|
|
if (isExiting) {
|
|
controller.set('error', null);
|
|
}
|
|
}
|
|
|
|
async beforeModel(transition) {
|
|
let exchangeOneTimeToken;
|
|
|
|
if (transition.to.queryParams.ott) {
|
|
exchangeOneTimeToken = this.get('token').exchangeOneTimeToken(transition.to.queryParams.ott);
|
|
} else {
|
|
exchangeOneTimeToken = Promise.resolve(true);
|
|
}
|
|
|
|
try {
|
|
await exchangeOneTimeToken;
|
|
} catch (e) {
|
|
this.controllerFor('application').set('error', e);
|
|
}
|
|
|
|
const fetchSelfTokenAndPolicies = this.get('token.fetchSelfTokenAndPolicies')
|
|
.perform()
|
|
.catch();
|
|
|
|
const fetchLicense = this.get('system.fetchLicense')
|
|
.perform()
|
|
.catch();
|
|
|
|
const promises = await RSVP.all([
|
|
this.get('system.regions'),
|
|
this.get('system.defaultRegion'),
|
|
fetchLicense,
|
|
fetchSelfTokenAndPolicies,
|
|
]);
|
|
|
|
if (!this.get('system.shouldShowRegions')) return promises;
|
|
|
|
const queryParam = transition.to.queryParams.region;
|
|
const defaultRegion = this.get('system.defaultRegion.region');
|
|
const currentRegion = this.get('system.activeRegion') || defaultRegion;
|
|
|
|
// Only reset the store if the region actually changed
|
|
if (
|
|
(queryParam && queryParam !== currentRegion) ||
|
|
(!queryParam && currentRegion !== defaultRegion)
|
|
) {
|
|
this.system.reset();
|
|
this.store.unloadAll();
|
|
}
|
|
|
|
this.set('system.activeRegion', queryParam || defaultRegion);
|
|
|
|
return promises;
|
|
}
|
|
|
|
// Model is being used as a way to propagate the region and
|
|
// one time token query parameters for use in setupController.
|
|
model({ region }, { to: { queryParams: { ott }}}) {
|
|
return {
|
|
region,
|
|
hasOneTimeToken: ott,
|
|
};
|
|
}
|
|
|
|
setupController(controller, { region, hasOneTimeToken }) {
|
|
if (region === this.get('system.defaultRegion.region')) {
|
|
next(() => {
|
|
controller.set('region', null);
|
|
});
|
|
}
|
|
|
|
super.setupController(...arguments);
|
|
|
|
if (hasOneTimeToken) {
|
|
// Hack to force clear the OTT query parameter
|
|
later(() => {
|
|
controller.set('oneTimeToken', '');
|
|
}, 500);
|
|
}
|
|
}
|
|
|
|
@action
|
|
didTransition() {
|
|
if (!this.get('config.isTest')) {
|
|
window.scrollTo(0, 0);
|
|
}
|
|
}
|
|
|
|
@action
|
|
willTransition() {
|
|
this.controllerFor('application').set('error', null);
|
|
}
|
|
|
|
@action
|
|
error(error) {
|
|
if (!(error instanceof AbortError)) {
|
|
this.controllerFor('application').set('error', error);
|
|
}
|
|
}
|
|
}
|