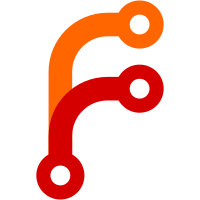
Clicking in a task group row in the job details page would throw the error: Uncaught Error: You didn't provide enough string/numeric parameters to satisfy all of the dynamic segments for route jobs.job.task-group. Missing params: name createParamHandlerInfo http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4814 applyToHandlers http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4804 applyToState http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4801 getTransitionByIntent http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4843 transitionByIntent http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4836 refresh http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4885 refresh http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:2254 queryParamsDidChange http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:2326 k http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:2423 triggerEvent http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:2349 fireQueryParamDidChange http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4863 getTransitionByIntent http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4848 transitionByIntent http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4836 doTransition http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4853 transitionTo http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:4882 _doTransition http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:2392 transitionTo http://localhost:4646/ui/assets/vendor-194b1e0d68d11ef7a4bf334eb30ba74d.js:2177 gotoTaskGroup http://localhost:4646/ui/assets/nomad-ui-4a2c1941e03e60e1feef715f23cf268c.js:623 ... This was caused because the attribute being passed to the transitionTo function was not the task group name, but the whole model.
86 lines
2.2 KiB
JavaScript
86 lines
2.2 KiB
JavaScript
import Component from '@ember/component';
|
|
import { inject as service } from '@ember/service';
|
|
import { computed, action } from '@ember/object';
|
|
import { alias, oneWay } from '@ember/object/computed';
|
|
import { debounce } from '@ember/runloop';
|
|
import {
|
|
classNames,
|
|
tagName,
|
|
attributeBindings,
|
|
} from '@ember-decorators/component';
|
|
import classic from 'ember-classic-decorator';
|
|
import { lazyClick } from '../helpers/lazy-click';
|
|
|
|
@classic
|
|
@tagName('tr')
|
|
@classNames('task-group-row', 'is-interactive')
|
|
@attributeBindings('data-test-task-group')
|
|
export default class TaskGroupRow extends Component {
|
|
@service can;
|
|
|
|
taskGroup = null;
|
|
debounce = 500;
|
|
|
|
@oneWay('taskGroup.count') count;
|
|
@alias('taskGroup.job.runningDeployment') runningDeployment;
|
|
|
|
get namespace() {
|
|
return this.get('taskGroup.job.namespace.name');
|
|
}
|
|
|
|
@computed('runningDeployment', 'namespace')
|
|
get tooltipText() {
|
|
if (this.can.cannot('scale job', null, { namespace: this.namespace }))
|
|
return "You aren't allowed to scale task groups";
|
|
if (this.runningDeployment)
|
|
return 'You cannot scale task groups during a deployment';
|
|
return undefined;
|
|
}
|
|
|
|
onClick() {}
|
|
|
|
click(event) {
|
|
lazyClick([this.onClick, event]);
|
|
}
|
|
|
|
@computed('count', 'taskGroup.scaling.min')
|
|
get isMinimum() {
|
|
const scaling = this.taskGroup.scaling;
|
|
if (!scaling || scaling.min == null) return false;
|
|
return this.count <= scaling.min;
|
|
}
|
|
|
|
@computed('count', 'taskGroup.scaling.max')
|
|
get isMaximum() {
|
|
const scaling = this.taskGroup.scaling;
|
|
if (!scaling || scaling.max == null) return false;
|
|
return this.count >= scaling.max;
|
|
}
|
|
|
|
@action
|
|
countUp() {
|
|
const scaling = this.taskGroup.scaling;
|
|
if (!scaling || scaling.max == null || this.count < scaling.max) {
|
|
this.incrementProperty('count');
|
|
this.scale(this.count);
|
|
}
|
|
}
|
|
|
|
@action
|
|
countDown() {
|
|
const scaling = this.taskGroup.scaling;
|
|
if (!scaling || scaling.min == null || this.count > scaling.min) {
|
|
this.decrementProperty('count');
|
|
this.scale(this.count);
|
|
}
|
|
}
|
|
|
|
scale(count) {
|
|
debounce(this, sendCountAction, count, this.debounce);
|
|
}
|
|
}
|
|
|
|
function sendCountAction(count) {
|
|
return this.taskGroup.scale(count);
|
|
}
|