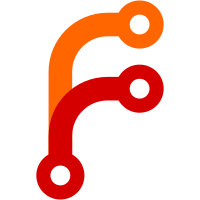
Nomad jobs may be configured with a TaskGroup which contains a Service definition that is Consul Connect enabled. These service definitions end up establishing a Consul Connect Proxy Task (e.g. envoy, by default). In the case where Consul ACLs are enabled, a Service Identity token is required for these tasks to run & connect, etc. This changeset enables the Nomad Server to recieve RPC requests for the derivation of SI tokens on behalf of instances of Consul Connect using Tasks. Those tokens are then relayed back to the requesting Client, which then injects the tokens in the secrets directory of the Task.
65 lines
1.5 KiB
Go
65 lines
1.5 KiB
Go
package structs
|
|
|
|
import "errors"
|
|
|
|
// An SIToken is the important bits of a Service Identity token generated by Consul.
|
|
type SIToken struct {
|
|
TaskName string
|
|
AccessorID string
|
|
SecretID string
|
|
}
|
|
|
|
// An SITokenAccessor is a reference to a created Service Identity token on
|
|
// behalf of an allocation's task.
|
|
type SITokenAccessor struct {
|
|
NodeID string
|
|
AllocID string
|
|
AccessorID string
|
|
TaskName string
|
|
|
|
// Raft index
|
|
CreateIndex uint64
|
|
}
|
|
|
|
// SITokenAccessorsRequest is used to operate on a set of SITokenAccessor, like
|
|
// recording a set of accessors for an alloc into raft.
|
|
type SITokenAccessorsRequest struct {
|
|
Accessors []*SITokenAccessor
|
|
}
|
|
|
|
// DeriveSITokenRequest is used to request Consul Service Identity tokens from
|
|
// the Nomad Server for the named tasks in the given allocation.
|
|
type DeriveSITokenRequest struct {
|
|
NodeID string
|
|
SecretID string
|
|
AllocID string
|
|
Tasks []string
|
|
QueryOptions
|
|
}
|
|
|
|
func (r *DeriveSITokenRequest) Validate() error {
|
|
switch {
|
|
case r.NodeID == "":
|
|
return errors.New("missing node ID")
|
|
case r.SecretID == "":
|
|
return errors.New("missing node SecretID")
|
|
case r.AllocID == "":
|
|
return errors.New("missing allocation ID")
|
|
case len(r.Tasks) == 0:
|
|
return errors.New("no tasks specified")
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
|
|
type DeriveSITokenResponse struct {
|
|
// Tokens maps from Task Name to its associated SI token
|
|
Tokens map[string]string
|
|
|
|
// Error stores any error that occurred. Errors are stored here so we can
|
|
// communicate whether it is retryable
|
|
Error *RecoverableError
|
|
|
|
QueryMeta
|
|
}
|