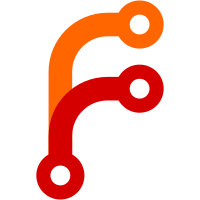
Previously, Nomad was using a hand-made lookup table for looking up EC2 CPU performance characteristics (core count + speed = ticks). This data was incomplete and incorrect depending on region. The AWS API has the correct data but requires API keys to use (i.e. should not be queried directly from Nomad). This change introduces a lookup table generated by a small command line tool in Nomad's tools module which uses the Amazon AWS API. Running the tool requires AWS_* environment variables set. $ # in nomad/tools/cpuinfo $ go run . Going forward, Nomad can incorporate regeneration of the lookup table somewhere in the CI pipeline so that we remain up-to-date on the latest offerings from EC2. Fixes #7830
49 lines
1.2 KiB
Plaintext
49 lines
1.2 KiB
Plaintext
// Code generated from hashicorp/nomad/tools/ec2info; DO NOT EDIT.
|
|
|
|
package {{.Package}}
|
|
|
|
// CPU contains virtual core count and processor baseline performance.
|
|
type CPU struct {
|
|
// use small units to reduce size of the embedded table
|
|
Cores uint32 // good for 4 billion cores
|
|
MHz uint32 // good for 4 billion MHz
|
|
}
|
|
|
|
// Ticks computes the total number of cycles available across the virtual
|
|
// cores of a CPU.
|
|
func (c CPU) Ticks() int {
|
|
return int(c.MHz) * int(c.Cores)
|
|
}
|
|
|
|
// GHz returns the speed of CPU in ghz.
|
|
func (c CPU) GHz() float64 {
|
|
return float64(c.MHz) / 1000.0
|
|
}
|
|
|
|
// newCPU create a CPUSpecs from the given virtual core count and core speed.
|
|
func newCPU(cores uint32, ghz float64) CPU {
|
|
return CPU{
|
|
Cores: cores,
|
|
MHz: uint32(ghz * 1000),
|
|
}
|
|
}
|
|
|
|
// LookupEC2CPU returns the virtual core count and core speed information from a
|
|
// lookup table generated from the Amazon EC2 API.
|
|
//
|
|
// If the instance type does not exist, nil is returned.
|
|
func LookupEC2CPU(instanceType string) *CPU {
|
|
specs, exists := instanceTypeCPU[instanceType]
|
|
if !exists {
|
|
return nil
|
|
}
|
|
return &specs
|
|
}
|
|
|
|
{{with .Data}}
|
|
var instanceTypeCPU = map[string]CPU {
|
|
{{ range $key, $value := . }}
|
|
"{{ $key }}": newCPU({{$value.Cores}}, {{$value.Speed}}), {{ end }}
|
|
}
|
|
{{end}}
|