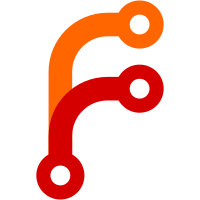
This PR fixes the artifact sandbox (new in Nomad 1.5) to allow downloading artifacts into the shared 'alloc' directory made available to each task in a common allocation. Previously we assumed the 'alloc' dir would be mounted under the 'task' dir, but this is only the case in fs isolation: chroot; in other modes the alloc dir is elsewhere.
38 lines
811 B
Go
38 lines
811 B
Go
//go:build windows
|
|
|
|
package getter
|
|
|
|
import (
|
|
"os"
|
|
"path/filepath"
|
|
"syscall"
|
|
)
|
|
|
|
// attributes returns the system process attributes to run
|
|
// the sandbox process with
|
|
func attributes() *syscall.SysProcAttr {
|
|
return &syscall.SysProcAttr{}
|
|
}
|
|
|
|
func credentials() (uint32, uint32) {
|
|
return 0, 0
|
|
}
|
|
|
|
// lockdown has no effect on windows
|
|
func lockdown(string, string) error {
|
|
return nil
|
|
}
|
|
|
|
// defaultEnvironment is the default minimal environment variables for Windows.
|
|
func defaultEnvironment(taskDir string) map[string]string {
|
|
tmpDir := filepath.Join(taskDir, "tmp")
|
|
return map[string]string{
|
|
"HOMEPATH": os.Getenv("HOMEPATH"),
|
|
"HOMEDRIVE": os.Getenv("HOMEDRIVE"),
|
|
"USERPROFILE": os.Getenv("USERPROFILE"),
|
|
"PATH": os.Getenv("PATH"),
|
|
"TMP": tmpDir,
|
|
"TEMP": tmpDir,
|
|
}
|
|
}
|