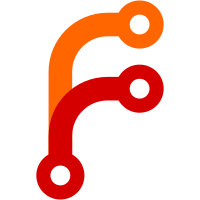
* client/executor: refactor client to remove interpolation * executor: POC libcontainer based executor * vendor: use hashicorp libcontainer fork * vendor: add libcontainer/nsenter dep * executor: updated executor interface to simplify operations * executor: implement logging pipe * logmon: new logmon plugin to manage task logs * driver/executor: use logmon for log management * executor: fix tests and windows build * executor: fix logging key names * executor: fix test failures * executor: add config field to toggle between using libcontainer and standard executors * logmon: use discover utility to discover nomad executable * executor: only call libcontainer-shim on main in linux * logmon: use seperate path configs for stdout/stderr fifos * executor: windows fixes * executor: created reusable pid stats collection utility that can be used in an executor * executor: update fifo.Open calls * executor: fix build * remove executor from docker driver * executor: Shutdown func to kill and cleanup executor and its children * executor: move linux specific universal executor funcs to seperate file * move logmon initialization to a task runner hook * client: doc fixes and renaming from code review * taskrunner: use shared config struct for logmon fifo fields * taskrunner: logmon only needs to be started once per task
55 lines
1.4 KiB
Go
55 lines
1.4 KiB
Go
package driver
|
|
|
|
import (
|
|
"io"
|
|
"net"
|
|
|
|
hclog "github.com/hashicorp/go-hclog"
|
|
"github.com/hashicorp/go-plugin"
|
|
)
|
|
|
|
var HandshakeConfig = plugin.HandshakeConfig{
|
|
ProtocolVersion: 1,
|
|
MagicCookieKey: "NOMAD_PLUGIN_MAGIC_COOKIE",
|
|
MagicCookieValue: "e4327c2e01eabfd75a8a67adb114fb34a757d57eee7728d857a8cec6e91a7255",
|
|
}
|
|
|
|
func GetPluginMap(w io.Writer, logLevel hclog.Level, fsIsolation bool) map[string]plugin.Plugin {
|
|
e := new(ExecutorPlugin)
|
|
|
|
e.logger = hclog.New(&hclog.LoggerOptions{
|
|
Output: w,
|
|
Level: logLevel,
|
|
})
|
|
|
|
e.fsIsolation = fsIsolation
|
|
|
|
return map[string]plugin.Plugin{
|
|
"executor": e,
|
|
}
|
|
}
|
|
|
|
// ExecutorReattachConfig is the config that we serialize and de-serialize and
|
|
// store in disk
|
|
type PluginReattachConfig struct {
|
|
Pid int
|
|
AddrNet string
|
|
AddrName string
|
|
}
|
|
|
|
// PluginConfig returns a config from an ExecutorReattachConfig
|
|
func (c *PluginReattachConfig) PluginConfig() *plugin.ReattachConfig {
|
|
var addr net.Addr
|
|
switch c.AddrNet {
|
|
case "unix", "unixgram", "unixpacket":
|
|
addr, _ = net.ResolveUnixAddr(c.AddrNet, c.AddrName)
|
|
case "tcp", "tcp4", "tcp6":
|
|
addr, _ = net.ResolveTCPAddr(c.AddrNet, c.AddrName)
|
|
}
|
|
return &plugin.ReattachConfig{Pid: c.Pid, Addr: addr}
|
|
}
|
|
|
|
func NewPluginReattachConfig(c *plugin.ReattachConfig) *PluginReattachConfig {
|
|
return &PluginReattachConfig{Pid: c.Pid, AddrNet: c.Addr.Network(), AddrName: c.Addr.String()}
|
|
}
|