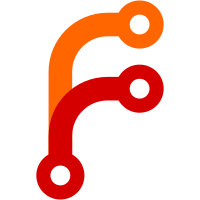
Adding '-verbose' will print out the allocation information for the deployment. This also changes the job run command so that it now blocks until deployment is complete and adds timestamps to the output so that it's more in line with the output of node drain. This uses glint to print in place in running in a tty. Because glint doesn't yet support cmd/powershell, Windows workflows use a different library to print in place, which results in slightly different formatting: 1) different margins, and 2) no spinner indicating deployment in progress.
76 lines
2 KiB
Go
76 lines
2 KiB
Go
package command
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/hashicorp/nomad/nomad/mock"
|
|
"github.com/mitchellh/cli"
|
|
"github.com/posener/complete"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestDeploymentStatusCommand_Implements(t *testing.T) {
|
|
t.Parallel()
|
|
var _ cli.Command = &DeploymentStatusCommand{}
|
|
}
|
|
|
|
func TestDeploymentStatusCommand_Fails(t *testing.T) {
|
|
t.Parallel()
|
|
ui := cli.NewMockUi()
|
|
cmd := &DeploymentStatusCommand{Meta: Meta{Ui: ui}}
|
|
|
|
// Fails on misuse
|
|
code := cmd.Run([]string{"some", "bad", "args"})
|
|
require.Equal(t, 1, code)
|
|
out := ui.ErrorWriter.String()
|
|
require.Contains(t, out, commandErrorText(cmd))
|
|
ui.ErrorWriter.Reset()
|
|
|
|
code = cmd.Run([]string{"-address=nope", "12"})
|
|
require.Equal(t, 1, code)
|
|
out = ui.ErrorWriter.String()
|
|
require.Contains(t, out, "Error retrieving deployment")
|
|
ui.ErrorWriter.Reset()
|
|
|
|
code = cmd.Run([]string{"-address=nope"})
|
|
require.Equal(t, 1, code)
|
|
out = ui.ErrorWriter.String()
|
|
// "deployments" indicates that we attempted to list all deployments
|
|
require.Contains(t, out, "Error retrieving deployments")
|
|
ui.ErrorWriter.Reset()
|
|
|
|
// Fails if monitor passed with json or tmpl flags
|
|
for _, flag := range []string{"-json", "-t"} {
|
|
code = cmd.Run([]string{"-monitor", flag, "12"})
|
|
require.Equal(t, 1, code)
|
|
out = ui.ErrorWriter.String()
|
|
require.Contains(t, out, "The monitor flag cannot be used with the '-json' or '-t' flags")
|
|
ui.ErrorWriter.Reset()
|
|
}
|
|
}
|
|
|
|
func TestDeploymentStatusCommand_AutocompleteArgs(t *testing.T) {
|
|
assert := assert.New(t)
|
|
t.Parallel()
|
|
|
|
srv, _, url := testServer(t, true, nil)
|
|
defer srv.Shutdown()
|
|
|
|
ui := cli.NewMockUi()
|
|
cmd := &DeploymentStatusCommand{Meta: Meta{Ui: ui, flagAddress: url}}
|
|
|
|
// Create a fake deployment
|
|
state := srv.Agent.Server().State()
|
|
d := mock.Deployment()
|
|
assert.Nil(state.UpsertDeployment(1000, d))
|
|
|
|
prefix := d.ID[:5]
|
|
args := complete.Args{Last: prefix}
|
|
predictor := cmd.AutocompleteArgs()
|
|
|
|
res := predictor.Predict(args)
|
|
assert.Equal(1, len(res))
|
|
assert.Equal(d.ID, res[0])
|
|
}
|