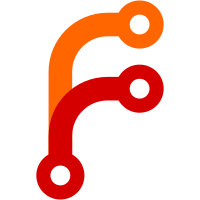
* Bones of a component that has job variable awareness * Got vars listed woo * Variables as its own subnav and some pathLinkedVariable perf fixes * Automatic Access to Variables alerter * Helper and component to conditionally render the right link * A bit of cleanup post-template stuff * testfix for looping right-arrow keynav bc we have a new subnav section * A very roundabout way of ensuring that, if a job exists when saving a variable with a pathLinkedEntity of that job, its saved right through to the job itself * hacky but an async version of pathLinkedVariable * model-driven and async fetcher driven with cleanup * Only run the update-job func if jobname is detected in var path * Test cases begun * Management token for variables to appear in tests * Its a management token so it gets to see the clients tab under system jobs * Pre-review cleanup * More tests * Number of requests test and small fix to groups-by-way-or-resource-arrays elsewhere * Variable intro text tests * Variable name re-use * Simplifying our wording a bit * parse json vs plainId * Addressed PR feedback, including de-waterfalling Co-authored-by: Phil Renaud <phil.renaud@hashicorp.com>
48 lines
1.2 KiB
JavaScript
48 lines
1.2 KiB
JavaScript
/**
|
|
* Copyright (c) HashiCorp, Inc.
|
|
* SPDX-License-Identifier: MPL-2.0
|
|
*/
|
|
|
|
// @ts-check
|
|
// eslint-disable-next-line no-unused-vars
|
|
import VariableModel from '../models/variable';
|
|
// eslint-disable-next-line no-unused-vars
|
|
import MutableArray from '@ember/array/mutable';
|
|
|
|
/**
|
|
* @typedef LinkToParams
|
|
* @property {string} route
|
|
* @property {string} model
|
|
* @property {Object} query
|
|
*/
|
|
|
|
import Helper from '@ember/component/helper';
|
|
|
|
/**
|
|
* Either generates a link to edit an existing variable, or else create a new one with a pre-filled path, depending on whether a variable with the given path already exists.
|
|
* Returns an object with route, model, and query; all strings.
|
|
* @param {Array<string>} positional
|
|
* @param {{ existingPaths: MutableArray<VariableModel>, namespace: string }} named
|
|
* @returns {LinkToParams}
|
|
*/
|
|
export function editableVariableLink(
|
|
[path],
|
|
{ existingPaths, namespace = 'default' }
|
|
) {
|
|
if (existingPaths.findBy('path', path)) {
|
|
return {
|
|
route: 'variables.variable.edit',
|
|
model: `${path}@${namespace}`,
|
|
query: {},
|
|
};
|
|
} else {
|
|
return {
|
|
route: 'variables.new',
|
|
model: '',
|
|
query: { path },
|
|
};
|
|
}
|
|
}
|
|
|
|
export default Helper.helper(editableVariableLink);
|