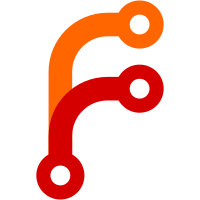
This can happen when one other node in the cluster such as a client is unable to communicate with the leader server and sees it as failed. When that happens its failing status eventually gets propagated to the other servers in the cluster and eventually this can result in RPCs returning “No cluster leader” error. That error is misleading and unhelpful for determing the root cause of the issue as its not raft stability but rather and client -> server networking issue. Therefore this commit will add a new error that will be returned in that case to differentiate between the two cases.
52 lines
1.8 KiB
Go
52 lines
1.8 KiB
Go
package structs
|
|
|
|
import (
|
|
"errors"
|
|
"strings"
|
|
)
|
|
|
|
const (
|
|
errNoLeader = "No cluster leader"
|
|
errNoDCPath = "No path to datacenter"
|
|
errDCNotAvailable = "Remote DC has no server currently reachable"
|
|
errNoServers = "No known Consul servers"
|
|
errNotReadyForConsistentReads = "Not ready to serve consistent reads"
|
|
errSegmentsNotSupported = "Network segments are not supported in this version of Consul"
|
|
errRPCRateExceeded = "RPC rate limit exceeded"
|
|
errServiceNotFound = "Service not found: "
|
|
errQueryNotFound = "Query not found"
|
|
errLeaderNotTracked = "Raft leader not found in server lookup mapping"
|
|
)
|
|
|
|
var (
|
|
ErrNoLeader = errors.New(errNoLeader)
|
|
ErrNoDCPath = errors.New(errNoDCPath)
|
|
ErrNoServers = errors.New(errNoServers)
|
|
ErrNotReadyForConsistentReads = errors.New(errNotReadyForConsistentReads)
|
|
ErrSegmentsNotSupported = errors.New(errSegmentsNotSupported)
|
|
ErrRPCRateExceeded = errors.New(errRPCRateExceeded)
|
|
ErrDCNotAvailable = errors.New(errDCNotAvailable)
|
|
ErrQueryNotFound = errors.New(errQueryNotFound)
|
|
ErrLeaderNotTracked = errors.New(errLeaderNotTracked)
|
|
)
|
|
|
|
func IsErrNoDCPath(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errNoDCPath)
|
|
}
|
|
|
|
func IsErrQueryNotFound(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errQueryNotFound)
|
|
}
|
|
|
|
func IsErrNoLeader(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errNoLeader)
|
|
}
|
|
|
|
func IsErrRPCRateExceeded(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errRPCRateExceeded)
|
|
}
|
|
|
|
func IsErrServiceNotFound(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errServiceNotFound)
|
|
}
|