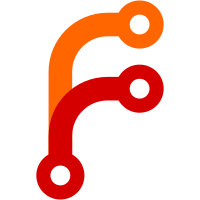
* ACL Authorizer overhaul To account for upcoming features every Authorization function can now take an extra *acl.EnterpriseAuthorizerContext. These are unused in OSS and will always be nil. Additionally the acl package has received some thorough refactoring to enable all of the extra Consul Enterprise specific authorizations including moving sentinel enforcement into the stubbed structs. The Authorizer funcs now return an acl.EnforcementDecision instead of a boolean. This improves the overall interface as it makes multiple Authorizers easily chainable as they now indicate whether they had an authoritative decision or should use some other defaults. A ChainedAuthorizer was added to handle this Authorizer enforcement chain and will never itself return a non-authoritative decision. * Include stub for extra enterprise rules in the global management policy * Allow for an upgrade of the global-management policy
73 lines
2 KiB
Go
73 lines
2 KiB
Go
package acl
|
|
|
|
import (
|
|
"errors"
|
|
"strings"
|
|
)
|
|
|
|
// These error constants define the standard ACL error types. The values
|
|
// must not be changed since the error values are sent via RPC calls
|
|
// from older clients and may not have the correct type.
|
|
const (
|
|
errNotFound = "ACL not found"
|
|
errRootDenied = "Cannot resolve root ACL"
|
|
errDisabled = "ACL support disabled"
|
|
errPermissionDenied = "Permission denied"
|
|
errInvalidParent = "Invalid Parent"
|
|
)
|
|
|
|
var (
|
|
// ErrNotFound indicates there is no matching ACL.
|
|
ErrNotFound = errors.New(errNotFound)
|
|
|
|
// ErrRootDenied is returned when attempting to resolve a root ACL.
|
|
ErrRootDenied = errors.New(errRootDenied)
|
|
|
|
// ErrDisabled is returned when ACL changes are not permitted since
|
|
// they are disabled.
|
|
ErrDisabled = errors.New(errDisabled)
|
|
|
|
// ErrPermissionDenied is returned when an ACL based rejection
|
|
// happens.
|
|
ErrPermissionDenied = PermissionDeniedError{}
|
|
|
|
// ErrInvalidParent is returned when a remotely resolve ACL
|
|
// token claims to have a non-root parent
|
|
ErrInvalidParent = errors.New(errInvalidParent)
|
|
)
|
|
|
|
// IsErrNotFound checks if the given error message is comparable to
|
|
// ErrNotFound.
|
|
func IsErrNotFound(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errNotFound)
|
|
}
|
|
|
|
// IsErrRootDenied checks if the given error message is comparable to
|
|
// ErrRootDenied.
|
|
func IsErrRootDenied(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errRootDenied)
|
|
}
|
|
|
|
// IsErrDisabled checks if the given error message is comparable to
|
|
// ErrDisabled.
|
|
func IsErrDisabled(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errDisabled)
|
|
}
|
|
|
|
// IsErrPermissionDenied checks if the given error message is comparable
|
|
// to ErrPermissionDenied.
|
|
func IsErrPermissionDenied(err error) bool {
|
|
return err != nil && strings.Contains(err.Error(), errPermissionDenied)
|
|
}
|
|
|
|
type PermissionDeniedError struct {
|
|
Cause string
|
|
}
|
|
|
|
func (e PermissionDeniedError) Error() string {
|
|
if e.Cause != "" {
|
|
return errPermissionDenied + ": " + e.Cause
|
|
}
|
|
return errPermissionDenied
|
|
}
|