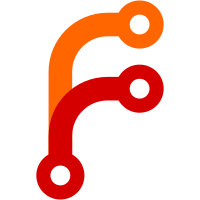
EventPublisher was receiving TopicHandlers, which had a couple of problems: - ChangeProcessors were being grouped by Topic, but they completely ignored the topic and were performed on every change - ChangeProcessors required EventPublisher to be aware of database changes By moving ChangeProcesors out of EventPublisher, and having Publish accept events instead of changes, EventPublisher no longer needs to be aware of these things. Handlers is now only SnapshotHandlers, which are still mapped by Topic. Also allows us to remove the small 'db' package that had only two types. They can now be unexported types in state.
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
/*
|
|
Package stream provides a publish/subscribe system for events produced by changes
|
|
to the state store.
|
|
*/
|
|
package stream
|
|
|
|
type Topic int32
|
|
|
|
// TODO: remove underscores
|
|
// TODO: type string instead of int?
|
|
// TODO: move topics to state package?
|
|
const (
|
|
Topic_ServiceHealth Topic = 1
|
|
Topic_ServiceHealthConnect Topic = 2
|
|
)
|
|
|
|
// TODO:
|
|
type Event struct {
|
|
Topic Topic
|
|
Key string
|
|
Index uint64
|
|
Payload interface{}
|
|
}
|
|
|
|
func (e Event) IsEndOfSnapshot() bool {
|
|
return e.Payload == endOfSnapshot{}
|
|
}
|
|
|
|
func (e Event) IsResumeStream() bool {
|
|
return e.Payload == resumeStream{}
|
|
}
|
|
|
|
type endOfSnapshot struct{}
|
|
|
|
type resumeStream struct{}
|
|
|
|
type closeSubscriptionPayload struct {
|
|
tokensSecretIDs []string
|
|
}
|
|
|
|
// NewCloseSubscriptionEvent returns a special Event that is handled by the
|
|
// stream package, and is never sent to subscribers. EventProcessor handles
|
|
// these events, and closes any subscriptions which were created using a token
|
|
// which matches any of the tokenSecretIDs.
|
|
func NewCloseSubscriptionEvent(tokenSecretIDs []string) Event {
|
|
return Event{Payload: closeSubscriptionPayload{tokensSecretIDs: tokenSecretIDs}}
|
|
}
|