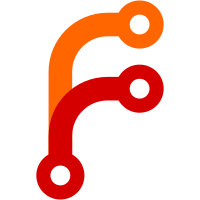
* Support for maximum size for Output of checks This PR allows users to limit the size of output produced by checks at the agent and check level. When set at the agent level, it will limit the output for all checks monitored by the agent. When set at the check level, it can override the agent max for a specific check but only if it is lower than the agent max. Default value is 4k, and input must be at least 1.
87 lines
2.5 KiB
Go
87 lines
2.5 KiB
Go
package structs
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/hashicorp/consul/api"
|
|
"github.com/hashicorp/consul/types"
|
|
)
|
|
|
|
// CheckDefinition is used to JSON decode the Check definitions
|
|
type CheckDefinition struct {
|
|
ID types.CheckID
|
|
Name string
|
|
Notes string
|
|
ServiceID string
|
|
Token string
|
|
Status string
|
|
|
|
// Copied fields from CheckType without the fields
|
|
// already present in CheckDefinition:
|
|
//
|
|
// ID (CheckID), Name, Status, Notes
|
|
//
|
|
ScriptArgs []string
|
|
HTTP string
|
|
Header map[string][]string
|
|
Method string
|
|
TCP string
|
|
Interval time.Duration
|
|
DockerContainerID string
|
|
Shell string
|
|
GRPC string
|
|
GRPCUseTLS bool
|
|
TLSSkipVerify bool
|
|
AliasNode string
|
|
AliasService string
|
|
Timeout time.Duration
|
|
TTL time.Duration
|
|
DeregisterCriticalServiceAfter time.Duration
|
|
OutputMaxSize int
|
|
}
|
|
|
|
func (c *CheckDefinition) HealthCheck(node string) *HealthCheck {
|
|
health := &HealthCheck{
|
|
Node: node,
|
|
CheckID: c.ID,
|
|
Name: c.Name,
|
|
Status: api.HealthCritical,
|
|
Notes: c.Notes,
|
|
ServiceID: c.ServiceID,
|
|
}
|
|
if c.Status != "" {
|
|
health.Status = c.Status
|
|
}
|
|
if health.CheckID == "" && health.Name != "" {
|
|
health.CheckID = types.CheckID(health.Name)
|
|
}
|
|
return health
|
|
}
|
|
|
|
func (c *CheckDefinition) CheckType() *CheckType {
|
|
return &CheckType{
|
|
CheckID: c.ID,
|
|
Name: c.Name,
|
|
Status: c.Status,
|
|
Notes: c.Notes,
|
|
|
|
ScriptArgs: c.ScriptArgs,
|
|
AliasNode: c.AliasNode,
|
|
AliasService: c.AliasService,
|
|
HTTP: c.HTTP,
|
|
GRPC: c.GRPC,
|
|
GRPCUseTLS: c.GRPCUseTLS,
|
|
Header: c.Header,
|
|
Method: c.Method,
|
|
OutputMaxSize: c.OutputMaxSize,
|
|
TCP: c.TCP,
|
|
Interval: c.Interval,
|
|
DockerContainerID: c.DockerContainerID,
|
|
Shell: c.Shell,
|
|
TLSSkipVerify: c.TLSSkipVerify,
|
|
Timeout: c.Timeout,
|
|
TTL: c.TTL,
|
|
DeregisterCriticalServiceAfter: c.DeregisterCriticalServiceAfter,
|
|
}
|
|
}
|