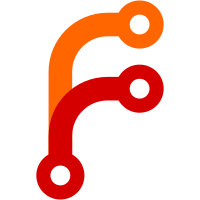
This patch removes duplicate internal copies of constants in the structs package which are also defined in the api package. The api.KVOp type with all its values for the TXN endpoint and the api.HealthXXX constants are now used throughout the codebase. This resulted in some circular dependencies in the testutil package which have been resolved by copying code and constants and moving the WaitForLeader function into a separate testrpc package.
62 lines
1 KiB
Go
62 lines
1 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"log"
|
|
"os"
|
|
|
|
"github.com/hashicorp/consul/lib"
|
|
"github.com/mitchellh/cli"
|
|
)
|
|
|
|
func init() {
|
|
lib.SeedMathRand()
|
|
}
|
|
|
|
func main() {
|
|
os.Exit(realMain())
|
|
}
|
|
|
|
func realMain() int {
|
|
log.SetOutput(ioutil.Discard)
|
|
|
|
// Get the command line args. We shortcut "--version" and "-v" to
|
|
// just show the version.
|
|
args := os.Args[1:]
|
|
for _, arg := range args {
|
|
if arg == "--" {
|
|
break
|
|
}
|
|
if arg == "-v" || arg == "--version" {
|
|
newArgs := make([]string, len(args)+1)
|
|
newArgs[0] = "version"
|
|
copy(newArgs[1:], args)
|
|
args = newArgs
|
|
break
|
|
}
|
|
}
|
|
|
|
// Filter out the configtest command from the help display
|
|
var included []string
|
|
for command := range Commands {
|
|
if command != "configtest" {
|
|
included = append(included, command)
|
|
}
|
|
}
|
|
|
|
cli := &cli.CLI{
|
|
Args: args,
|
|
Commands: Commands,
|
|
HelpFunc: cli.FilteredHelpFunc(included, cli.BasicHelpFunc("consul")),
|
|
}
|
|
|
|
exitCode, err := cli.Run()
|
|
if err != nil {
|
|
fmt.Fprintf(os.Stderr, "Error executing CLI: %s\n", err.Error())
|
|
return 1
|
|
}
|
|
|
|
return exitCode
|
|
}
|