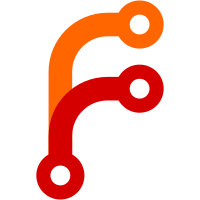
In #2191 I accedentally broke SCADA by not populating the agent's version information into the config structure. This adds it back, and makes the distinction between the raw parts we send to APIs and the human form of the version that we display.
44 lines
1 KiB
Go
44 lines
1 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
// The git commit that was compiled. This will be filled in by the compiler.
|
|
var (
|
|
GitCommit string
|
|
GitDescribe string
|
|
)
|
|
|
|
// The main version number that is being run at the moment.
|
|
const Version = "0.7.0"
|
|
|
|
// A pre-release marker for the version. If this is "" (empty string)
|
|
// then it means that it is a final release. Otherwise, this is a pre-release
|
|
// such as "dev" (in development), "beta", "rc1", etc.
|
|
const VersionPrerelease = "dev"
|
|
|
|
// GetHumanVersion composes the parts of the version in a way that's suitable
|
|
// for displaying to humans.
|
|
func GetHumanVersion() string {
|
|
version := Version
|
|
if GitDescribe != "" {
|
|
version = GitDescribe
|
|
}
|
|
|
|
release := VersionPrerelease
|
|
if GitDescribe == "" && release == "" {
|
|
release = "dev"
|
|
}
|
|
if release != "" {
|
|
version += fmt.Sprintf("-%s", release)
|
|
if GitCommit != "" {
|
|
version += fmt.Sprintf(" (%s)", GitCommit)
|
|
}
|
|
}
|
|
|
|
// Strip off any single quotes added by the git information.
|
|
return strings.Replace(version, "'", "", -1)
|
|
}
|