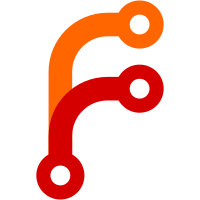
* Add data layer for discovery chain (model/adapter/serializer/repo) * Add routing plus template for routing tab * Add extra deps - consul-api-double upgrade plus ngraph for graphing * Add discovery-chain and related components and helpers: 1. discovery-chain to orchestrate/view controller 2. route-card, splitter-card, resolver card to represent the 3 different node types. 3. route-match helper for easy formatting of route rules 4. dom-position to figure out where things are in order to draw lines 5. svg-curve, simple wrapper around svg's <path d=""> attribute format. 6. data-structs service. This isn't super required but we are using other data-structures provided by other third party npm modules in other yet to be merged PRs. All of these types of things will live here for easy access/injection/changability 7. Some additions to our css-var 'polyfill' for a couple of extra needed rules * Related CSS for discovery chain 1. We add a %card base component here, eventually this will go into our base folder and %stats-card will also use it for a base component. 2. New icon for failovers * ui: Discovery Chain Continued (#6939) 1. Add in the things we use for the animations 2 Use IntersectionObserver so we know when the tab is visible, otherwise the dom-position helper won't work as the dom elements don't have any display. 3. Add some base work for animations and use them a little 4. Try to detect if a resolver is a redirect. Right now this works for datacenters and namespaces, but it can't work for services and subsets - we are awaiting backend support for doing this properly. 5. Add a fake 'this service has no routes' route that says 'Default' 6. redirect icon 7. Add CSS.escape polyfill for Edge
115 lines
3.7 KiB
JavaScript
115 lines
3.7 KiB
JavaScript
import Service from '@ember/service';
|
|
import { getOwner } from '@ember/application';
|
|
import { guidFor } from '@ember/object/internals';
|
|
|
|
// selecting
|
|
import qsaFactory from 'consul-ui/utils/dom/qsa-factory';
|
|
// TODO: sibling and closest seem to have 'PHP-like' guess the order arguments
|
|
// ie. one `string, element` and the other has `element, string`
|
|
// see if its possible to standardize
|
|
import sibling from 'consul-ui/utils/dom/sibling';
|
|
import closest from 'consul-ui/utils/dom/closest';
|
|
import isOutside from 'consul-ui/utils/dom/is-outside';
|
|
import getComponentFactory from 'consul-ui/utils/dom/get-component-factory';
|
|
|
|
// events
|
|
import normalizeEvent from 'consul-ui/utils/dom/normalize-event';
|
|
import createListeners from 'consul-ui/utils/dom/create-listeners';
|
|
import clickFirstAnchorFactory from 'consul-ui/utils/dom/click-first-anchor';
|
|
|
|
// ember-eslint doesn't like you using a single $ so use double
|
|
// use $_ for components
|
|
const $$ = qsaFactory();
|
|
let $_;
|
|
let inViewportCallbacks;
|
|
const clickFirstAnchor = clickFirstAnchorFactory(closest);
|
|
export default Service.extend({
|
|
doc: document,
|
|
win: window,
|
|
init: function() {
|
|
this._super(...arguments);
|
|
inViewportCallbacks = new WeakMap();
|
|
$_ = getComponentFactory(getOwner(this));
|
|
},
|
|
document: function() {
|
|
return this.doc;
|
|
},
|
|
viewport: function() {
|
|
return this.win;
|
|
},
|
|
guid: function(el) {
|
|
return guidFor(el);
|
|
},
|
|
// TODO: should this be here? Needs a better name at least
|
|
clickFirstAnchor: clickFirstAnchor,
|
|
closest: closest,
|
|
sibling: sibling,
|
|
isOutside: isOutside,
|
|
normalizeEvent: normalizeEvent,
|
|
listeners: createListeners,
|
|
root: function() {
|
|
return this.doc.documentElement;
|
|
},
|
|
// TODO: Should I change these to use the standard names
|
|
// even though they don't have a standard signature (querySelector*)
|
|
elementById: function(id) {
|
|
return this.doc.getElementById(id);
|
|
},
|
|
elementsByTagName: function(name, context) {
|
|
context = typeof context === 'undefined' ? this.doc : context;
|
|
return context.getElementsByTagName(name);
|
|
},
|
|
elements: function(selector, context) {
|
|
// don't ever be tempted to [...$$()] here
|
|
// it should return a NodeList
|
|
return $$(selector, context);
|
|
},
|
|
element: function(selector, context) {
|
|
if (selector.substr(0, 1) === '#') {
|
|
return this.elementById(selector.substr(1));
|
|
}
|
|
// TODO: This can just use querySelector
|
|
return [...$$(selector, context)][0];
|
|
},
|
|
// ember components aren't strictly 'dom-like'
|
|
// but if you think of them as a web component 'shim'
|
|
// then it makes more sense to think of them as part of the dom
|
|
// with traditional/standard web components you wouldn't actually need this
|
|
// method as you could just get to their methods from the dom element
|
|
component: function(selector, context) {
|
|
if (typeof selector !== 'string') {
|
|
return $_(selector);
|
|
}
|
|
return $_(this.element(selector, context));
|
|
},
|
|
components: function(selector, context) {
|
|
return [...this.elements(selector, context)]
|
|
.map(function(item) {
|
|
return $_(item);
|
|
})
|
|
.filter(function(item) {
|
|
return item != null;
|
|
});
|
|
},
|
|
isInViewport: function($el, cb, threshold = 0) {
|
|
inViewportCallbacks.set($el, cb);
|
|
const observer = new IntersectionObserver(
|
|
(entries, observer) => {
|
|
entries.map(item => {
|
|
const cb = inViewportCallbacks.get(item.target);
|
|
if (typeof cb === 'function') {
|
|
cb(item.isIntersecting);
|
|
}
|
|
});
|
|
},
|
|
{
|
|
rootMargin: '0px',
|
|
threshold: threshold,
|
|
}
|
|
);
|
|
observer.observe($el); // eslint-disable-line ember/no-observers
|
|
// observer.unobserve($el);
|
|
return () => observer.disconnect(); // eslint-disable-line ember/no-observers
|
|
},
|
|
});
|