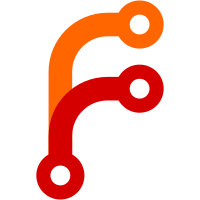
Now that testutil uses t.Cleanup to remove the directory the caller no longer has to manage the removal
137 lines
2.6 KiB
Go
137 lines
2.6 KiB
Go
package rolelist
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/hashicorp/consul/agent"
|
|
"github.com/hashicorp/consul/api"
|
|
"github.com/hashicorp/consul/testrpc"
|
|
"github.com/mitchellh/cli"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestRoleListCommand_noTabs(t *testing.T) {
|
|
t.Parallel()
|
|
|
|
if strings.ContainsRune(New(cli.NewMockUi()).Help(), '\t') {
|
|
t.Fatal("help has tabs")
|
|
}
|
|
}
|
|
|
|
func TestRoleListCommand(t *testing.T) {
|
|
t.Parallel()
|
|
require := require.New(t)
|
|
|
|
a := agent.NewTestAgent(t, `
|
|
primary_datacenter = "dc1"
|
|
acl {
|
|
enabled = true
|
|
tokens {
|
|
master = "root"
|
|
}
|
|
}`)
|
|
|
|
defer a.Shutdown()
|
|
testrpc.WaitForLeader(t, a.RPC, "dc1")
|
|
|
|
ui := cli.NewMockUi()
|
|
cmd := New(ui)
|
|
|
|
var roleIDs []string
|
|
|
|
// Create a couple roles to list
|
|
client := a.Client()
|
|
svcids := []*api.ACLServiceIdentity{
|
|
{ServiceName: "fake"},
|
|
}
|
|
for i := 0; i < 5; i++ {
|
|
name := fmt.Sprintf("test-role-%d", i)
|
|
|
|
role, _, err := client.ACL().RoleCreate(
|
|
&api.ACLRole{Name: name, ServiceIdentities: svcids},
|
|
&api.WriteOptions{Token: "root"},
|
|
)
|
|
roleIDs = append(roleIDs, role.ID)
|
|
|
|
require.NoError(err)
|
|
}
|
|
|
|
args := []string{
|
|
"-http-addr=" + a.HTTPAddr(),
|
|
"-token=root",
|
|
}
|
|
|
|
code := cmd.Run(args)
|
|
require.Equal(code, 0)
|
|
require.Empty(ui.ErrorWriter.String())
|
|
output := ui.OutputWriter.String()
|
|
|
|
for i, v := range roleIDs {
|
|
require.Contains(output, fmt.Sprintf("test-role-%d", i))
|
|
require.Contains(output, v)
|
|
}
|
|
}
|
|
|
|
func TestRoleListCommand_JSON(t *testing.T) {
|
|
t.Parallel()
|
|
require := require.New(t)
|
|
|
|
a := agent.NewTestAgent(t, `
|
|
primary_datacenter = "dc1"
|
|
acl {
|
|
enabled = true
|
|
tokens {
|
|
master = "root"
|
|
}
|
|
}`)
|
|
|
|
defer a.Shutdown()
|
|
testrpc.WaitForLeader(t, a.RPC, "dc1")
|
|
|
|
ui := cli.NewMockUi()
|
|
cmd := New(ui)
|
|
|
|
var roleIDs []string
|
|
|
|
// Create a couple roles to list
|
|
client := a.Client()
|
|
svcids := []*api.ACLServiceIdentity{
|
|
{ServiceName: "fake"},
|
|
}
|
|
for i := 0; i < 5; i++ {
|
|
name := fmt.Sprintf("test-role-%d", i)
|
|
|
|
role, _, err := client.ACL().RoleCreate(
|
|
&api.ACLRole{Name: name, ServiceIdentities: svcids},
|
|
&api.WriteOptions{Token: "root"},
|
|
)
|
|
roleIDs = append(roleIDs, role.ID)
|
|
|
|
require.NoError(err)
|
|
}
|
|
|
|
args := []string{
|
|
"-http-addr=" + a.HTTPAddr(),
|
|
"-token=root",
|
|
"-format=json",
|
|
}
|
|
|
|
code := cmd.Run(args)
|
|
require.Equal(code, 0)
|
|
require.Empty(ui.ErrorWriter.String())
|
|
output := ui.OutputWriter.String()
|
|
|
|
for i, v := range roleIDs {
|
|
require.Contains(output, fmt.Sprintf("test-role-%d", i))
|
|
require.Contains(output, v)
|
|
}
|
|
|
|
var jsonOutput json.RawMessage
|
|
err := json.Unmarshal([]byte(output), &jsonOutput)
|
|
assert.NoError(t, err)
|
|
}
|