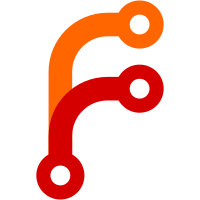
This PR removes storybook and adds docfy and uses docfy to render our existing README files. This now means we can keep adding README documentation without committing any specific format or framework. If we eventually move to storybook then fine, or if we just want to remove docfy for whatever reason then fine - we will still have a full set of README files viewable via GitHub.
69 lines
2.2 KiB
JavaScript
69 lines
2.2 KiB
JavaScript
/* eslint-env node */
|
|
/*eslint node/no-extraneous-require: "off"*/
|
|
'use strict';
|
|
//
|
|
const $ = process.env;
|
|
const fs = require('fs');
|
|
const path = require('path');
|
|
const promisify = require('util').promisify;
|
|
const read = promisify(fs.readFile);
|
|
const apiDouble = require('@hashicorp/api-double');
|
|
|
|
const mergeTrees = require('broccoli-merge-trees');
|
|
const writeFile = require('broccoli-file-creator');
|
|
|
|
const apiDoubleHeaders = require('@hashicorp/api-double/lib/headers');
|
|
const cookieParser = require('cookie-parser');
|
|
const bodyParser = require('body-parser');
|
|
|
|
//
|
|
module.exports = {
|
|
name: 'startup',
|
|
serverMiddleware: function(server) {
|
|
// TODO: see if we can move these into the project specific `/server` directory
|
|
// instead of inside an addon
|
|
|
|
// TODO: This should all be moved out into ember-cli-api-double
|
|
// and we should figure out a way to get to the settings here for
|
|
// so we can set this path name centrally in config
|
|
// TODO: undefined here is a possible faker salt that we should be able
|
|
// to pass in from ember serve/config somehow
|
|
const dir = path.resolve('./mock-api');
|
|
const controller = apiDouble(undefined, dir, read, $, path.resolve);
|
|
[
|
|
apiDoubleHeaders(),
|
|
cookieParser(),
|
|
bodyParser.text({ type: '*/*' }),
|
|
controller().serve,
|
|
].reduce(function(app, item) {
|
|
return app.use(item);
|
|
}, server.app);
|
|
},
|
|
treeFor: function(name) {
|
|
const tree = this._super.treeFor.apply(this, arguments);
|
|
if (name === 'app') {
|
|
const prodlike = ['production', 'staging'];
|
|
if (prodlike.includes(process.env.EMBER_ENV)) {
|
|
return mergeTrees([tree, writeFile('components/debug/navigation/index.hbs', '')]);
|
|
}
|
|
}
|
|
return tree;
|
|
},
|
|
contentFor: function(type, config) {
|
|
const vars = {
|
|
appName: config.modulePrefix,
|
|
environment: config.environment,
|
|
rootURL: config.environment === 'production' ? '{{.ContentPath}}' : config.rootURL,
|
|
config: config,
|
|
};
|
|
switch (type) {
|
|
case 'head':
|
|
return require('./templates/head.html.js')(vars);
|
|
case 'body':
|
|
return require('./templates/body.html.js')(vars);
|
|
case 'root-class':
|
|
return 'ember-loading';
|
|
}
|
|
},
|
|
};
|